rails 调用接口
Rails is great when implementing CRUD for our models, (Create, Read, Update, and Destroy). It is well structured for the developer to be in control of the data that the application has to offer and to what access allows for the user on what to do with such data.
在为我们的模型实现CRUD(创建,读取,更新和销毁)时,Rails很棒。 开发人员可以很好地控制应用程序必须提供的数据以及对用户的访问权限,以控制该数据的处理方式。
Using the controllers and defining the methods in there grants the user the flexibility to do all these actions, but in order to see the changes, the page needs to reload, and there is nothing wrong with that, but it will be nicer if we can make these actions more dynamic and allow the application to behave more like other sites where there is no need for reloading the page is truly beneficial.
使用控制器并在其中定义方法可以使用户灵活地执行所有这些操作,但是要查看更改,需要重新加载页面,这样做没有什么问题,但是如果可以的话,会更好。使这些动作更具动态性,并使应用程序的行为更像其他不需要重新加载页面的站点,这确实是有益的。
As I dive into javascript, I am learning the use of functions and events, and there is a new concept I am exploring called Ajax calls.
在学习javascript时,我正在学习函数和事件的使用,并且正在探索一个新概念,称为Ajax调用。
AJAX, based on w3schools refers to ‘Asynchronous JavaScript And XML’. Ajax allows web pages to be updated asynchronously by exchanging data with a web server behind the scenes. This means that it is possible to update parts of a web page without reloading.
基于w3schools的AJAX指的是“异步JavaScript和XML”。 Ajax允许通过与后台的Web服务器交换数据来异步更新网页。 这意味着可以更新网页的某些部分而无需重新加载。
AJAX如何运作 (How AJAX Works)
I will be using the above method in this blog to call on the delete action on my application and to delete the selected data without the need of reloading the page.
我将在此博客中使用以上方法在我的应用程序上调用delete操作并删除选定的数据,而无需重新加载页面。
One way to delete an instance of a class without reloading the page.
一种无需重新加载页面即可删除类实例的方法。
For this mechanism to work, the idea is to communicate with the back-end via javascript on the front-end. The server is responsible for generating the JSON code to respond to the client on the front-end.
为了使这种机制有效,其想法是通过前端的javascript与后端进行通信。 服务器负责生成JSON代码以响应前端上的客户端。
Rails can make use of JSON Response using the concept of remote true, this is enabled by default in rails, and it will reduce the amount of Js code for the action to work.
Rails可以使用remote true的概念使用JSON Response,默认情况下在Rails中启用了它,它将减少Js代码的数量以使操作正常进行。
#/app/contollers/students_controller.rb
class StudentsController < ApplicationController ...def destroy
@student = Student.find(params[:id])
@student.destroy #It will dictate what format the action will respond to respond_to do |format|
format.html# when responding to a html request, it will respond by going into /app/views/students/destroy.html.erb format.js
#when responding to a json request, it will respond by generating js code located in /app/views/students/destroy.js.erb end
end ...
end
It all depends on how we set the respond_to, on which method, and what is the end goal for the call.
这完全取决于我们如何设置response_to,采用哪种方法以及调用的最终目标是什么。
# /app/views/instructors/index.js.erb
let instructorsUl = document.querySelector("ul#instructors")
# Ruby code will get executed, and this will communicate with the front-end via javascript. <% @instructors.each do |instructor| %>
instructorLi = document.createElement("li")
instructorLi.id = "instructor-<%= instructor.id %>"
instructorLi.innerText = "<%= instructor.first_name %>"
instructorsUl.append(instructorLi)
<% end %>
At this moment my application students#index looks like this
此时,我的应用程序students#index看起来像这样
# /app/views/students/index.html.erb<% @students.map do |student_info| %>
<tbody id="deleteStudent<%= student_info.id %>">
<tr class="text-center"> <br>
<td class="text-center"><%= link_to student_info.id, student_path(student_info), :class => 'btn btn-default' %></td>
<td><%= student_info.name.capitalize %></td>
<td><%= student_info.language %></td>
<td> <%= student_info.age %> </td>
<td><%= link_to(student_path(student_info), method: :delete, remote: true) do %>
<p class="text-center"><span class="glyphicon glyphicon-trash"></span></p>
<% end %>
</td>
</tr>
</tbody>
<% end %>
Above, the bold lines of code are in charge of communicating what is happening in the back-end to the front-end, in order words, when I delete a student instance, I need the front-end to reflect such change without the need for the user to reload the page.
上面,粗体代码行负责将后端发生的事情传达到前端,按顺序说,当我删除一个学生实例时,我需要前端来反映这种变化而无需供用户重新加载页面。
For that to work, I need to create the logic in the destroy.js.erb that I call on from the Student controller.
为此,我需要在从Student控制器调用的destroy.js.erb中创建逻辑。
# /app/views/students/destroy.js.erblet delete<%= @student.id %> = document.querySelector("#deleteStudent" + <%= @student.id %>);delete<%= @student.id %>.remove();
By having the action of destroy defined in the controller, I am allowing the user once they clicked on the button, to make the request for the delete action. As explained by Erin “when adding rails helper remote: true this stops the default action of the object (known as preventDefault() in Javascript) and instead performs the Ajax call. You are still required to provide a path that points to the appropriate action corresponding to the correct HTTP request.”
通过在控制器中定义销毁操作,我允许用户单击按钮后就可以请求删除操作。 如Erin所述: “在添加rails helper remote时:true会停止对象的默认操作(在Javascript中称为preventDefault() ),而是执行Ajax调用。 您仍然需要提供一条路径,该路径指向与正确的HTTP请求相对应的适当操作。”
Once the appropriate path has been given, the action goes to the controller method and looks for the view where is it been sent to, in this case, it is going to be rendered to format.js which points to the destroy.js.erb. This view is telling the application to look for an id tag of deleteStudent that has the student.id attached to it, and once it finds it, it is telling it to remove it.
给定适当的路径后,操作将转到控制器方法并查找将其发送到的视图,在这种情况下,它将呈现为format.js ,该格式指向destroy.js.erb。 。 该视图告诉应用程序寻找一个附加了student.id的deleteStudent ID标记,一旦找到它,便告诉它删除它。
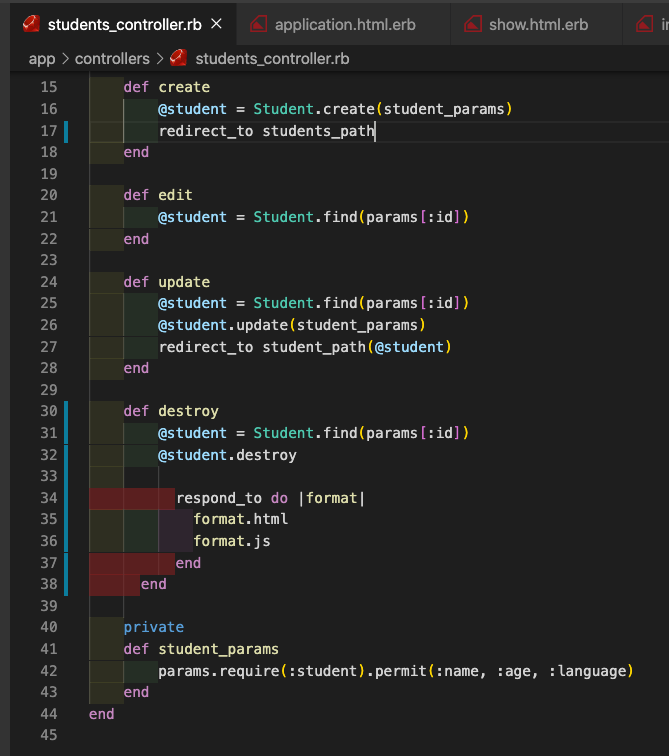
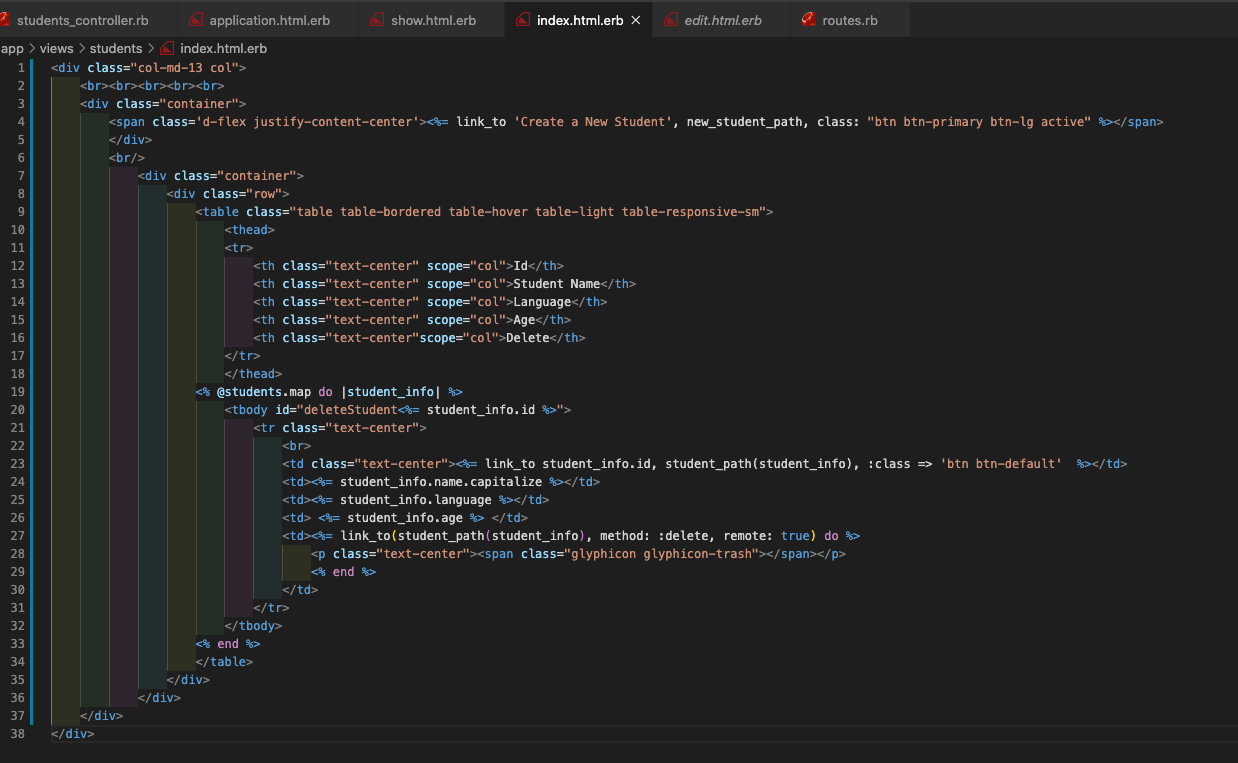
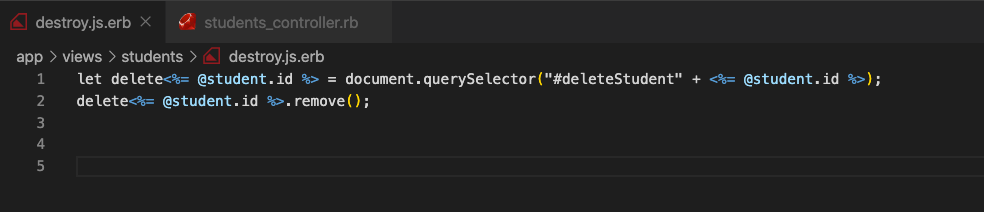
结论 (Conclusion)
The delete action still depends on the controller and the method destroy, what I have accomplished here by adding the Ajax call, is for this action to occur without the need of reloading the page. Without the destroy.js.erb the student instance still gets deleted from the database, but for the user to see such change, the page needs to be reloaded. With destroy.js.erb the user experience is enhanced by removing the extra step.
删除操作仍然取决于控制器和销毁方法,而我通过添加Ajax调用在此处完成的目的是使此操作发生而无需重新加载页面。 如果没有destroy.js.erb,学生实例仍然会从数据库中删除,但是为了让用户看到这样的更改,需要重新加载页面。 使用destroy.js.erb,可以消除多余的步骤,从而提高了用户体验。
演示地址
资源资源(Resources)
翻译自: https://medium.com/@fbado66/ajax-calls-in-rails-delete-actions-without-reloading-the-page-934fc1b4b9cd
rails 调用接口