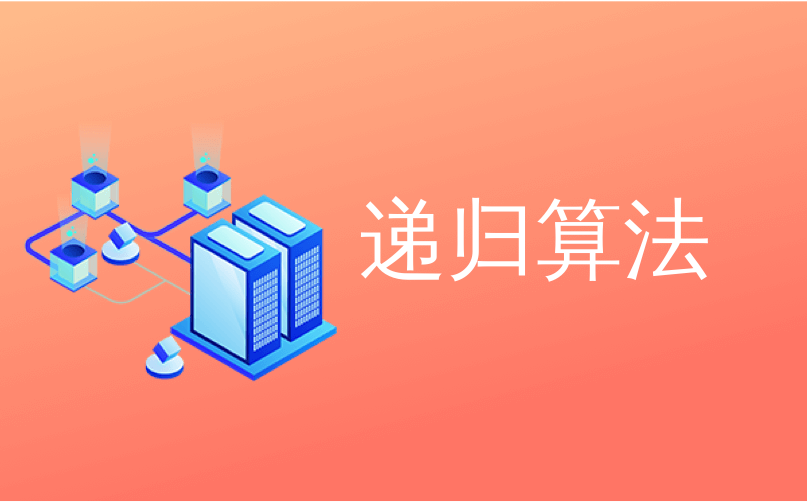
递归算法
抽象
是否曾经需要分解WAR文件以及分解WAR文件中的所有JAR文件? 是的,我也是!
我写了ferris-war-exploder来爆炸:
- 一个JAR文件
- 一个WAR文件,它找到的每个JAR文件也会爆炸。
- 包含每个JAR文件(请参阅#1)和WAR文件(请参阅#2)的EAR文件也爆炸了。
基本上,ferris-war-exploder会爆炸任何ZIP文件格式的东西。 ZIP格式的所有条目也会被分解。 这是递归发生的,因此任何可以爆炸的东西都会爆炸。
免责声明
这篇文章仅供参考。 在使用所提供的任何信息之前,请认真思考。 从中学到东西,但最终自己做出决定,风险自负。
要求
我使用以下主要技术完成了本文的所有工作。 您可能可以使用不同的技术或版本来做相同的事情,但不能保证。
- NetBeans 11.2
- Maven 3.3.9(与NetBeans捆绑在一起)
- Java 11(zulu11.35.15-ca-jdk11.0.5-win_x64)
下载
访问我的GitHub页面https://github.com/mjremijan以查看我所有的开源项目。 这篇文章的代码位于: https : //github.com/mjremijan/ferris-war-exploder
让我们开始吧
ferris-war-exploder会爆炸任何ZIP文件格式的文件。 ZIP格式的所有条目也会被分解。 这是递归发生的,因此任何可以爆炸的东西都会爆炸。
您需要告诉它要爆炸的档案(WAR,JAR,EAR,ZIP)。
您需要告诉它在哪里爆炸存档。
注意一旦WAR爆炸,请参阅我的ferris-magic-number来分析所有.class
文件。
清单1显示了启动应用程序的main()
方法。 我有2个示例:爆炸一个JAR和爆炸一个WAR。
清单1 –
public class Main {
public static void main(String[] args) throws Exception
{
System.out.printf( "=== Welcome to Ferris WAR Exploder ===%n" );
new Unzip( "./src/test/jars/commons-lang3-3.7.jar" , "./target/unzipped/jar" )
.unzip();
new Unzip( "./src/test/wars/sample.war" , "./target/unzipped/war" )
.unzip();
System.out.printf( "%n=== DONE ===%n" );
}
}
清单2显示了Unzip
类。 此类包含有趣的代码以递归方式分解档案。 清单2中的所有内容都不难理解,因此请您自己仔细阅读。
清单2 –
package org.ferris.war.exploder;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.zip.ZipEntry;
import java.util.zip.ZipFile;
import java.util.zip.ZipInputStream;
/**
*
* @author Michael Remijan mjremijan@yahoo.com @mjremijan
*/
public class Unzip {
protected File zipFile;
protected File destinationDirectory;
public Unzip(String zipFilePath, String destinationDirectoryPath) {
setZipFile(zipFilePath);
setDestinationDirectory(destinationDirectoryPath);
}
public Unzip(File zipFile) {
this .zipFile = zipFile;
setDestinationDirectory(zipFile.getParent());
}
protected void setDestinationDirectory(String destinationDirectoryPath) {
destinationDirectory = new File(destinationDirectoryPath, zipFile.getName());
if (destinationDirectory.exists() && destinationDirectory.isDirectory()) {
throw new RuntimeException(
String.format(
"The destination directory \"%s\" already exists." ,
destinationDirectory.getPath()
)
);
}
if (destinationDirectory.exists() && destinationDirectory.isFile()) {
destinationDirectory = new File(destinationDirectoryPath, zipFile.getName() + ".d" );
}
mkdirs(destinationDirectory,
"Failed to create the destination directory \"%s\"."
);
}
protected void setZipFile(String zipFilePath) {
zipFile = new File(zipFilePath);
if (!zipFile.exists()) {
throw new RuntimeException(
String.format(
"The file \"%s\" does not exist" , zipFile.getPath()
)
);
}
if (!zipFile.canRead()) {
throw new RuntimeException(
String.format(
"The file \"%s\" is not readable" , zipFile.getPath()
)
);
}
}
protected void unzip() throws Exception {
System.out.printf( "%n=== Unipping %s ===%n%n" , zipFile.getPath());
try (ZipInputStream zip
= new ZipInputStream( new FileInputStream(zipFile));
){
for (ZipEntry z = zip.getNextEntry(); z != null ; z = zip.getNextEntry()) {
if (z.isDirectory()) {
mkdirs( new File(destinationDirectory, z.getName()),
"Failed to create a zip entry directory \"%s\""
);
} else {
File zfile = new File(destinationDirectory, z.getName());
mkdirs(zfile.getParentFile(),
"Failed to create parent directory for zip entry file \"%s\"."
);
File unzippedFile = unzipEntry(z, zip);
if (isZip(unzippedFile)) {
new Unzip(unzippedFile).unzip();
}
}
}
}
}
protected boolean isZip(File file) {
boolean b = false ;
try {
b = new ZipFile(file).getName().length() > 0 ;
} catch (IOException ignore) {}
return b;
}
protected File unzipEntry(ZipEntry z, ZipInputStream zip) throws Exception {
File zfile = new File(destinationDirectory, z.getName());
System.out.printf( " %s%n" , zfile.getAbsolutePath());
try ( FileOutputStream out = new FileOutputStream(zfile)) {
zip.transferTo(out);
}
zip.closeEntry();;
return zfile;
}
protected void mkdirs(File dir, String errorMessageFormat) {
if (dir.exists() && dir.isDirectory()) {
return ;
}
dir.mkdirs();
if (!dir.exists()) {
throw new RuntimeException(
String.format(errorMessageFormat, dir.getPath()
)
);
}
}
}
概要
ferris-war-exploder项目并不太复杂,但是当您需要完全爆炸WAR或EAR归档文件时,它非常方便。 请享用!
参考文献
ZipOutputStream。 (nd)。 Oracle从https://docs.oracle.com/en/java/javase/11/docs/api/java.base/java/util/zip/ZipOutputStream.html检索。
翻译自: https://www.javacodegeeks.com/2020/03/explode-a-war-file-recursively.html
递归算法