ef延迟加载和非延迟加载
By default, Angular loads all modules as soon as the web application starts up, whether they are needed or not is irrelevant. As an Angular application grows and more modules and components get added, the size of the compiled bundle (HTML, CSS, and Typescript) generated by Angular increases.
默认情况下,Angular会在Web应用程序启动后立即加载所有模块,无论是否需要它们都是无关紧要的。 随着Angular应用程序的增长和更多模块和组件的添加,Angular生成的已编译捆绑包(HTML,CSS和Typescript)的大小会增加。
The bundle size gets bigger, and as a direct correlation, the amount of time it takes to load the web application also increases. End-users may not necessarily notice the difference, but, you may want to implement Lazy Loading in your Angular application.
捆绑包的大小会变大,并且作为直接关联,加载Web应用程序所花费的时间也会增加。 最终用户不一定会注意到差异,但是,您可能希望在Angular应用程序中实现延迟加载。
什么是延迟加载 (What is Lazy Loading)
Instead of loading the whole compiled bundle, which takes time and network resources, lazy loading allows for the Angular web app to be split into multiple smaller, and manageable chunks. These chunks are then only loaded when needed.
延迟加载无需将整个编译后的捆绑包加载在一起(这会花费时间和网络资源),而是可以将Angular Web应用程序拆分为多个较小的可管理块。 然后仅在需要时加载这些块。
延迟加载的优点 (Advantages of lazy loading)
Fewer network resources loaded: When a user loads the web app, instead of all resources of that application being downloaded, a module/chunk will be downloaded and rendered based on the content being requested. This is also helpful on mobile devices especially when an end-user could be outside of a Wi-Fi hotspot.
加载的网络资源更少:当用户加载Web应用程序时,不是下载该应用程序的所有资源,而是根据请求的内容下载并渲染模块/块。 这在移动设备上也很有用,特别是当最终用户可能不在Wi-Fi热点之外时。
A decrease in loading time: According to a study conducted by Google in 2018, if a page loads between 1 to 3 seconds, the probability of a user leaving the website increases to 32%. Lazy loading a webpage reduces bundle size, allowing for a quicker loading time.
加载时间的减少:根据Google在2018年进行的一项研究 ,如果页面加载时间在1-3秒之间,则用户离开网站的可能性会增加到32%。 延迟加载网页可减小包的大小,从而缩短加载时间。
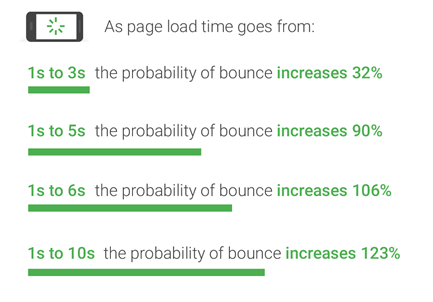
在Angular中实现延迟加载 (Implementing Lazy Loading in Angular)
To demonstrate lazy-loading, I created a simple Angular project containing three components: about-us, contact-us, and home. The following steps will demonstrate how to create the components as well as their modules.
为了演示延迟加载,我创建了一个简单的Angular项目,其中包含三个组件:about-us,contact-us和home。 以下步骤将演示如何创建组件及其模块。
生成组件 (Generating the components)
Create a component called home
. Run this command in your console.
创建一个名为home
的组件。 在控制台中运行此命令。
ng g c home
ng gc home
Create a contact-us
component as well as its module to be used for routing and lazy loading.
创建一个contact-us
组件及其模块,以用于路由和延迟加载。
ng g c contact-us
ng gc contact-us
Creating the contact-us
module with routing.
使用路由创建contact-us
模块。
ng g m contact-us –-routing
ng gm contact-us –-routing
The same thing to be done for the about-us
component.
about-us
组件要执行相同的操作。
ng g c about-us
ng gc about-us
ng g m about-us –-routing
ng gm about-us –-routing
The home component will be a runtime loaded component. On the other hand, the two lazily loaded components will be about-us and contact-us.
home组件将是运行时加载的组件。 另一方面,两个延迟加载的组件将是“大约使用”和“接触使用”。
Now that we have three empty components, let’s add some basic styling and add routes. Thereafter we are going to create a simple nav-bar that allows us to route to different components.
现在我们有了三个空组件,让我们添加一些基本样式并添加路线。 此后,我们将创建一个简单的导航栏,该导航栏允许我们路由到不同的组件。
In the src/app/app.component.html
file, add the following code :
在src/app/app.component.html
文件中,添加以下代码:
In the src/app/app.component.scss
add the following CSS:
在src/app/app.component.scss
添加以下CSS:
Go back to your browser, and now we have three links that take us to different components. Now, let’s add some routing so that we can see the components that are loaded when the links in the nav-bar are clicked.
回到您的浏览器,现在我们有了三个链接,可将我们带到不同的组件。 现在,让我们添加一些路由,以便在单击导航栏中的链接时可以看到加载的组件。
In the routes array in src/app/app-routing.module.ts
, add the following paths. Don’t forget to import the components.
在src/app/app-routing.module.ts
的路由数组中,添加以下路径。 不要忘记导入组件。
关于我们的组件 (About-us component)
Add the following HTML to src/app/about-us/about-us.component.html
将以下HTML添加到src/app/about-us/about-us.component.html
Now add some styling to the component.
现在,向组件添加一些样式。
Now, we add a route in the about-us module which takes us to the about-us component. Make sure to also import your component.
现在,我们在about-us模块中添加一条路线,该路线将我们带到about-us组件。 确保还导入您的组件。
You should see this as a result if you click on the About link in the nav-bar.
如果单击导航栏中的关于链接,您应该会看到这样的结果。
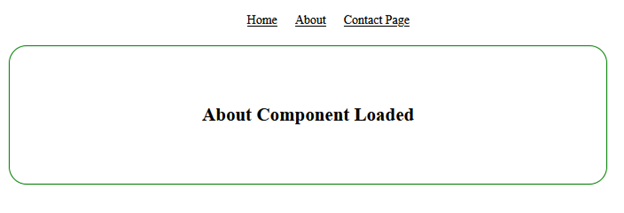
联系我们组件 (Contact-us component)
Add the following HTML and styling to src/app/contact-us/
将以下HTML和样式添加到src/app/contact-us/
Now, we add a route in the contact-us module which takes us to the contact-us component. Make sure to also import your component.
现在,我们在contact-us模块中添加一条路线,将我们带到contact-us组件。 确保还导入您的组件。
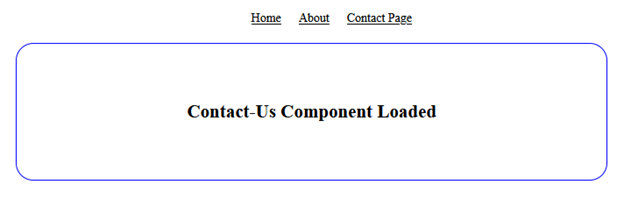
家庭组件 (Home component)
The home component will not be lazy-loaded for demonstration purposes so there is no module for it needed. Add some HTML and styling in src/app/home/
出于演示目的,不会延迟加载home组件,因此不需要模块。 在src/app/home/
添加一些HTML和样式
And finally, you should see this if the home component is loaded correctly.
最后,如果正确加载了home组件,则应该看到此信息。
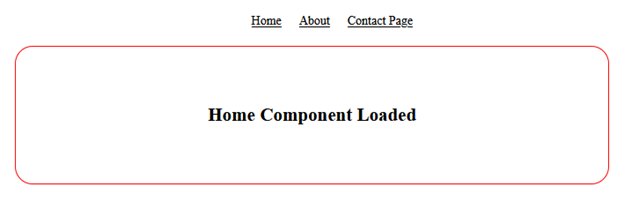
Now, we are not finished yet as these components are not loaded separately when clicked.
现在,我们还没有完成,因为单击时这些组件没有单独加载。
让我们添加一些延迟加载! (Let’s add some lazy loading!)
At the beginning of the tutorial, where we added our routes to the various components, we specified a certain component to be showed once a link is clicked. Now instead of loading a component, we are going to be loading a module that contains routes and paths of a specific component.
在本教程的开始,我们将路由添加到各个组件中,我们指定了一个特定组件,一旦单击链接,该组件将显示。 现在,我们将加载包含特定组件的路由和路径的模块,而不是加载组件。
We are going to use a loadChildren
property in the path. This property uses an arrow function which is called to resolve and locate a collection of lazy-loaded routes of a specific component. Let’s update the routes array in the app-routing.module.ts
file.
我们将在路径中使用loadChildren
属性。 此属性使用箭头函数,该函数被调用来解析和定位特定组件的延迟加载路由的集合。 让我们更新app-routing.module.ts
文件中的路由数组。
Loading modules with the loadChildren
property
使用loadChildren
属性加载模块
Go back to your browser and click on the home component link. Go to the developer tools, click on the Network tab. You can see that the runtime.js, polyfills.js, styles.js, vendor.js, and main.js files are initially loaded. These are code bundles generated by Angular.
返回浏览器,然后单击home组件链接。 转到开发人员工具,单击“网络”选项卡。 您可以看到runtime.js,polyfills.js,styles.js,vendor.js和main.js文件是最初加载的。 这些是Angular生成的代码包。
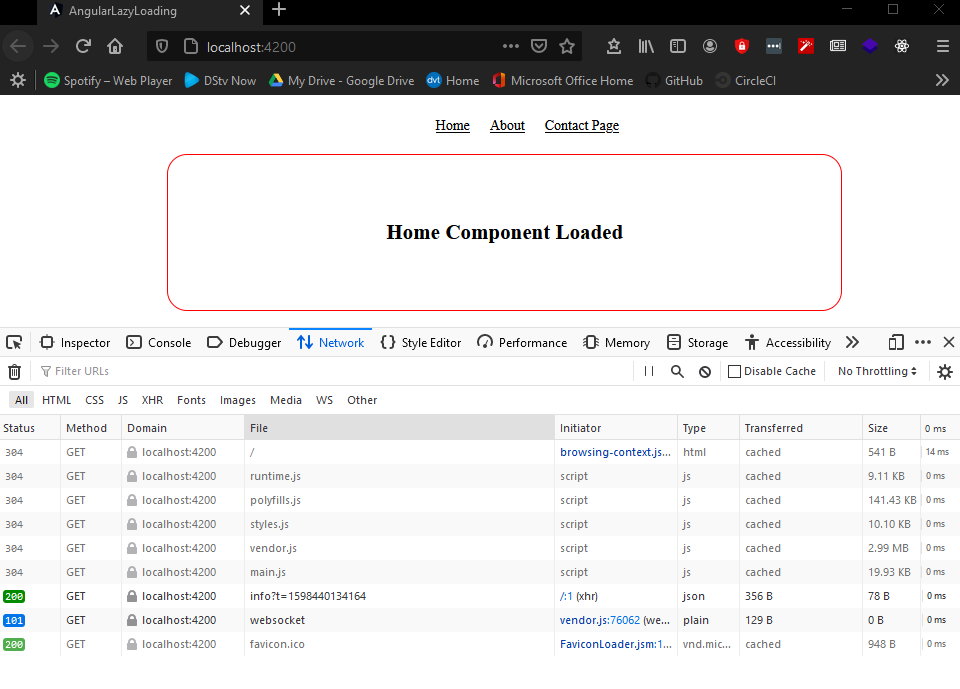
Once you click on the About link or the Contact Page link, you will firstly notice that the About component is loaded, but also you will see within the network tab, the About-Us chunk is loaded.
单击“关于”链接或“联系页面”链接后,您首先会注意到已加载“关于”组件,但也会在网络选项卡中看到“关于-Us”块已加载。
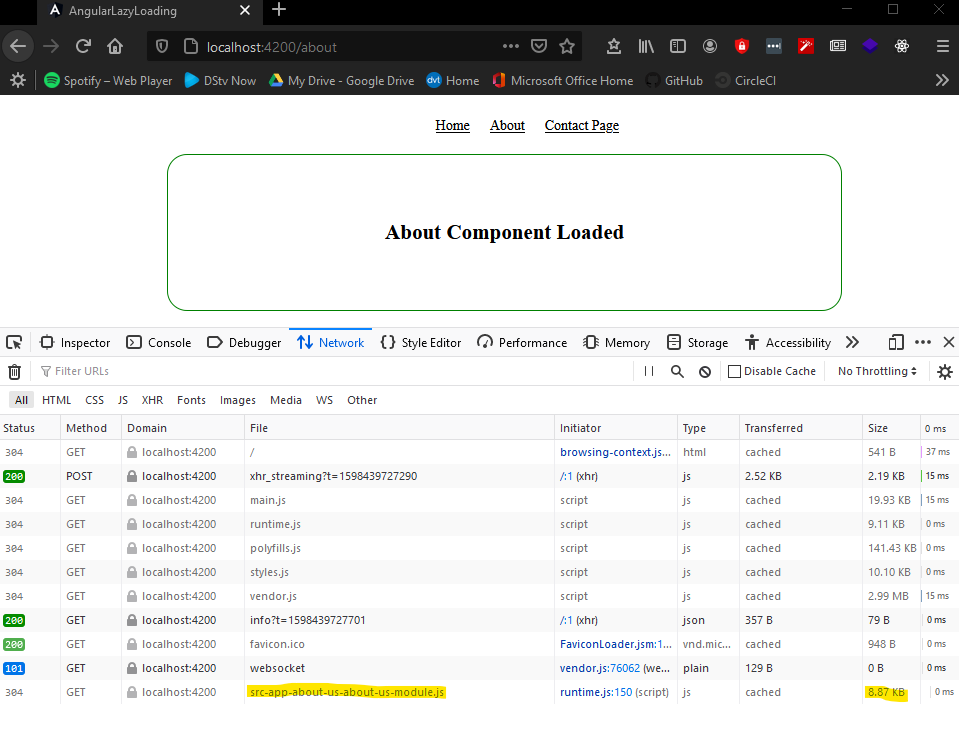
Next, click on the Contact Page link.
接下来,单击“联系页面”链接。
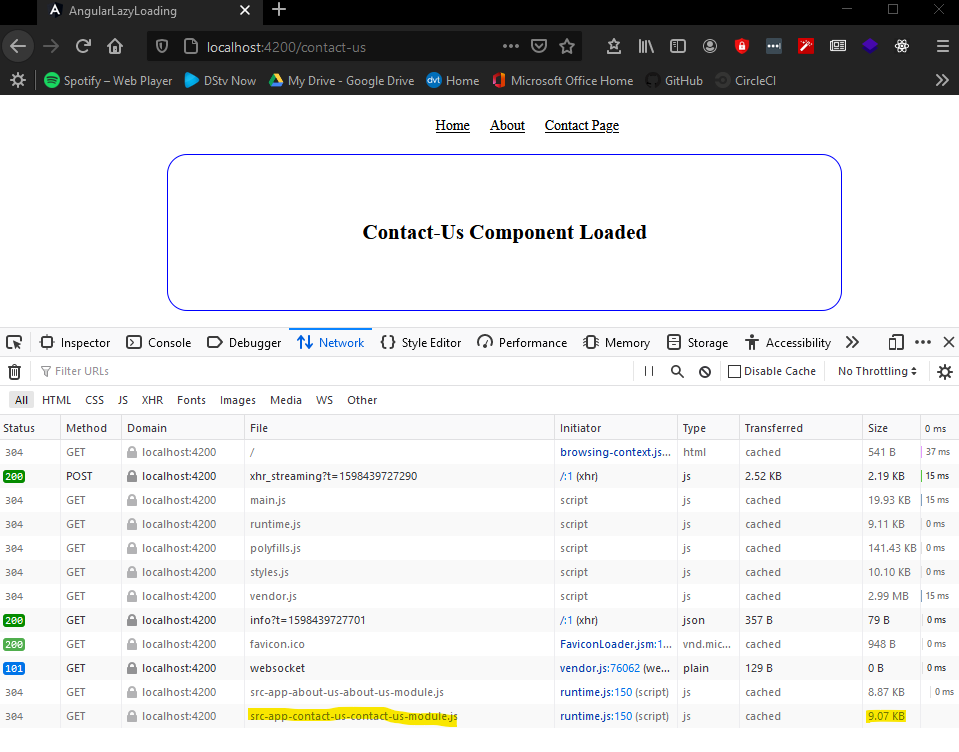
Now, clicking on the About link again, will not load any module because the module has already been loaded. Notice how small the size of the chunks are.
现在,再次单击关于链接,将不会加载任何模块,因为该模块已经加载。 注意块的大小。
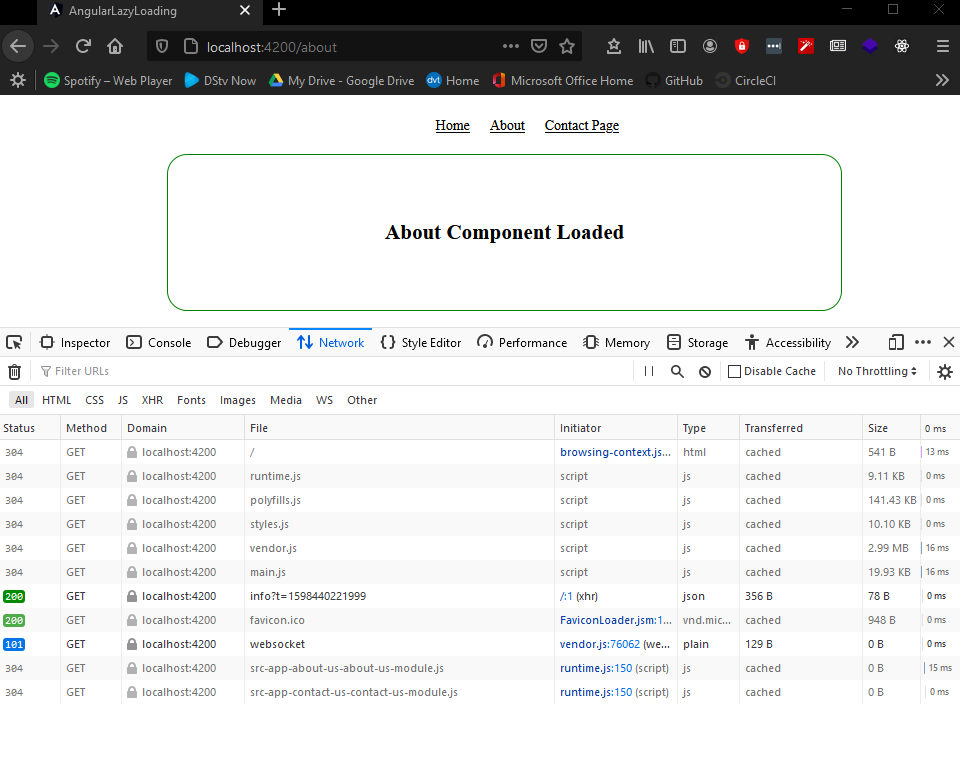
结论 (In conclusion)
Separating an application into modules/chunks is a good way to improve the loading time of an Angular application, as well as to keep the file size of the application low.
将应用程序划分为模块/块是改善Angular应用程序的加载时间以及使应用程序的文件大小保持较小的好方法。
If you have any problems with the code above, here is a link to the Github repository which contains the final code.
如果您对以上代码有任何疑问,请访问 Github存储库,其中包含最终代码。
查看其他资源 (See other resources)
https://ultimatecourses.com/blog/lazy-load-angular-modules
https://ultimatecourses.com/blog/lazy-load-angular-modules
Thanks to DVT and Mr Kennedy Sigauke, developer at DVT for the proofreading.
感谢DVT和DVT开发人员Kennedy Sigauke先生的校对。
翻译自: https://medium.com/dvt-engineering/angular-lazy-loading-simple-but-effective-f891c288557b
ef延迟加载和非延迟加载