背景 (Background)
After reading Vasudha Mamtani’s blog about sign-up pages, I realized I had been taking them for granted.
阅读了Vasudha Mamtani关于注册页面的博客后,我意识到我已经把它们视为理所当然了。
Having a solid sign-up page affects whether or not someone uses your website. Would you enter your email into a wonky-looking sign-up form?
拥有可靠的注册页面会影响是否有人使用您的网站。 您是否可以将电子邮件输入看上去有点奇怪的注册表格?
After looking through the examples in Mamtani’s blog, I decided to try my hand at making a better sign-in page. I came up with this:
浏览完Mamtani博客中的示例后,我决定尝试制作一个更好的登录页面。 我想出了这个 :
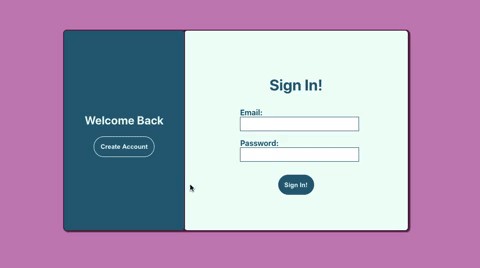
The plan here is for a user to only see the abstract image when switching between signing in and creating an account.
这里的计划是让用户仅在登录和创建帐户之间切换时才能看到抽象图像。
I’m trying to imply that there’s a complicated inner working to this website. We are capable of great and complicated things. Trust us!
我试图暗示这个网站的内部工作很复杂。 我们有能力做伟大而复杂的事情。 相信我们!
讲解 (Tutorial)
In the following, I’ll explain how to code one of these sign-up pages using React and CSS.
在下文中,我将解释如何使用React和CSS编写这些注册页面之一。
Link to GitHub
链接到GitHub
目录 (Table of Contents)
- Preliminary Junk 初步垃圾
- Container Layout 容器布局
- Banner and Form Transitions 标语和表格过渡
- Conditionally Render Sign-In / Sign-Up 有条件地渲染登录/注册
- Conclusion 结论
初步垃圾 (Preliminary Junk)
First, as has become ritual for these blogs, I installed a create-react-app
, deleted default stuff, and set up file structure and components.
首先,作为这些博客的惯例,我安装了create-react-app
,删除了默认内容,并设置了文件结构和组件。
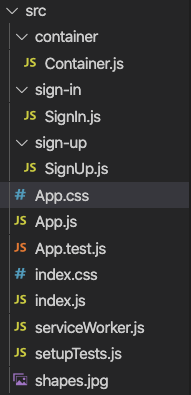
As you can see, there are three main components. Container.js
is my outermost element. Inside it, there are two divs for the form side and the banner side.
如您所见,有三个主要组成部分。 Container.js
是我最外面的元素。 在其中,表单面和横幅面有两个div。
Then, there are two form components for whether you are signing in or creating a new account, SignIn.js
and SignUp.js
respectively. These are conditionally rendered on the form side of Container.js
.
然后,有两个表单组件分别用于登录或创建新帐户,分别为SignIn.js
和SignUp.js
。 它们有条件地呈现在Container.js
的表单侧。
I added a CSS class called cfb
(center flexbox). To reduce repetition, whenever I need contents of a div centered, I throw on a cfb
. As needed, I add other flex-related properties, like flex-direction
, to the specific element.
我添加了一个名为cfb
(中央flexbox)CSS类。 为了减少重复,每当我需要以div为中心的内容时,我都会抛出cfb
。 根据需要,我向特定元素添加了其他与flex相关的属性,例如flex-direction
。

容器布局 (Container Layout)
Container div
容器div
I style the main div in Container.js
like this:
我在Container.js
设置主div的样式如下:
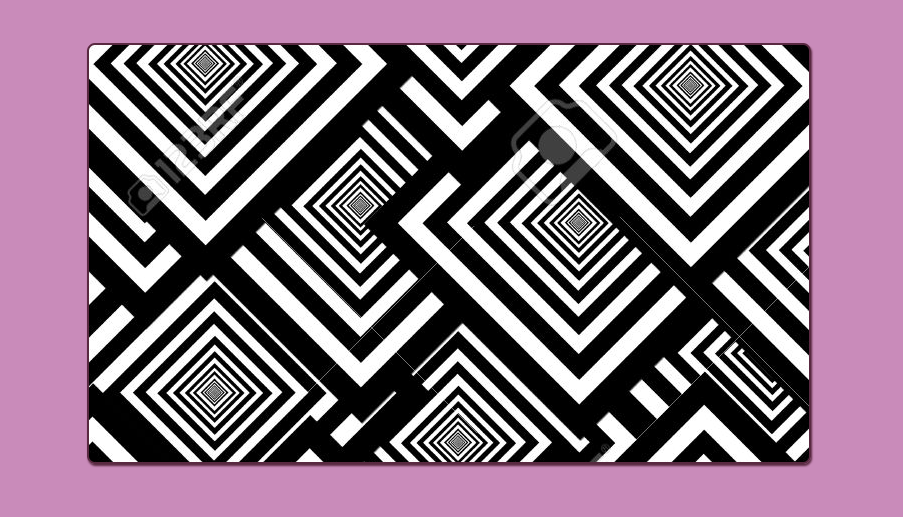
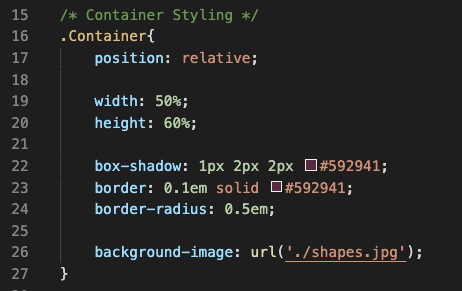
Form Side and Banner Side
表格面和横幅面
Next, I create divs for the banner side and form side of Container.js
.
接下来,我为Container.js
的横幅面和表单面创建div。
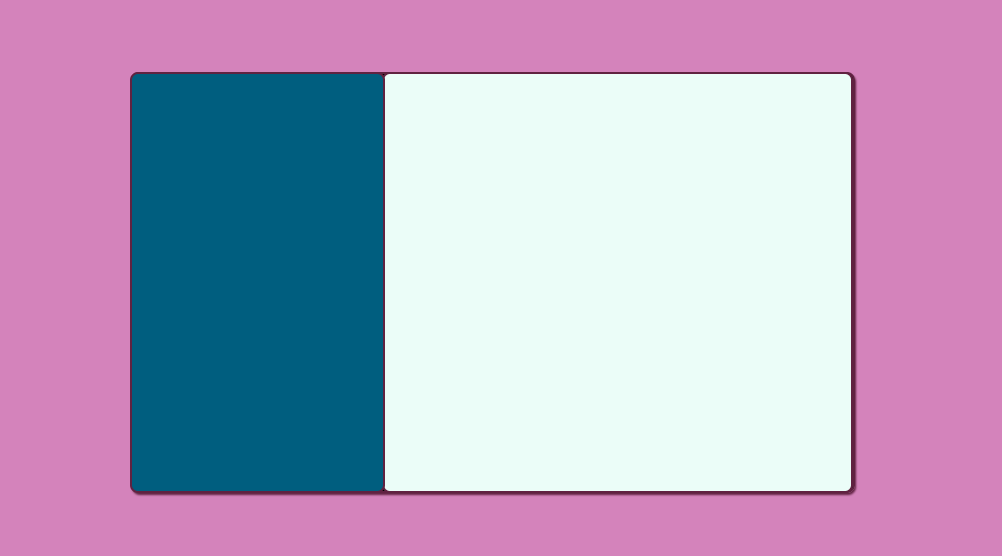
Form:
形成:
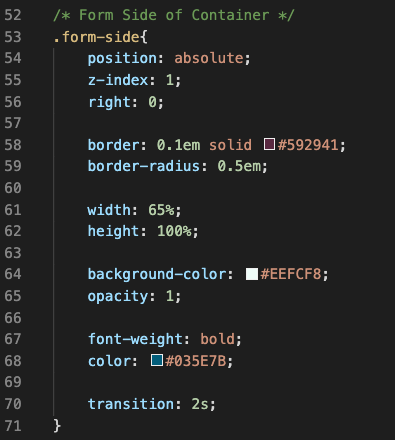
Banner:
旗帜:
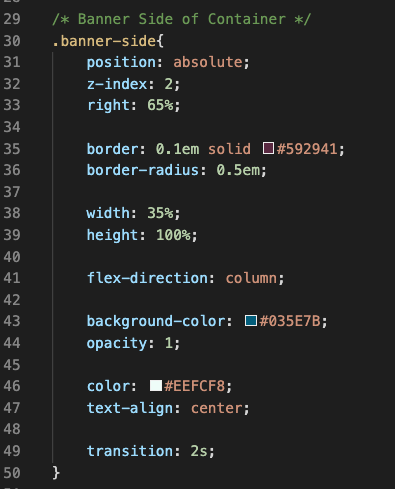
标语和表格过渡 (Banner and Form Transitions)
Positioning
定位方式
So the transitions work properly, I set up CSS properties for position
, right
, z-index
, and transition
.
因此过渡效果正常,我为position
, right
, z-index
和transition
设置了CSS属性。
To make the divs free to move, they must be styled with a position: absolute;
. Then, they will align themselves within the nearest positioned parent element. This means I also must style the main div in Container.js
to have a position: relative;
.
为了使div自由移动,必须为它们设置以下position: absolute;
。 然后,它们将在最靠近的父元素内对齐。 这意味着我还必须在Container.js
设置主div的样式,使其具有以下position: relative;
。
I’ve styled the size of the divs with percentages. I use these same sizes to set how far right
they should be.
我已经用百分比设置了div的大小。 我用这些相同的尺寸,以一套多远right
,他们应该是。
First, the form-side
is set at right: 0;
. It will sit flush on the right side of the container.
首先,将form-side
设置为right: 0;
。 它会齐平地位于容器的右侧。
Because the form-side
is 65%
of the parent element, I set the banner-side
to be right: 65%;
. It starts where the form-side
ends.
因为form-side
是父元素的65%
,所以我将banner-side
设置为right: 65%;
。 它从form-side
结束的地方开始。
To get banner-side
to hover over form-side
, I give banner-side
a z-index: 2;
and the form-side
a z-index: 1;
.
为了使banner-side
悬停在form-side
,我给banner-side
一个z-index: 2;
以及form-side
的z-index: 1;
。
Finally, I give them each a transition: 2s;
. When I change their right
properties, they will move fluidly from their starting place to the next for the duration of two seconds.
最后,我给他们一个transition: 2s;
。 当我更改其right
属性时,它们将在两秒钟的时间内从起始位置流畅地移动到下一个位置。
Triggering Transitions by Updating Classes
通过更新类触发转换
The positioning of banner-side
and form-side
will be based on whether I am rendering a sign-in or sign-up form.
banner-side
和form-side
将取决于我是呈现登录表单还是注册表单。
I set up two new classes for where the banner-side
and form-side
will be when they are reversed.
我设置了两个新的类,分别用于反转banner-side
和form-side
。
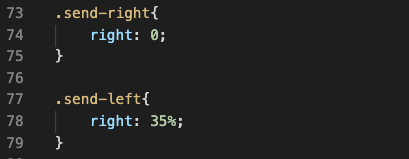
I apply these new classes with functions.
我将这些新类与函数一起应用。
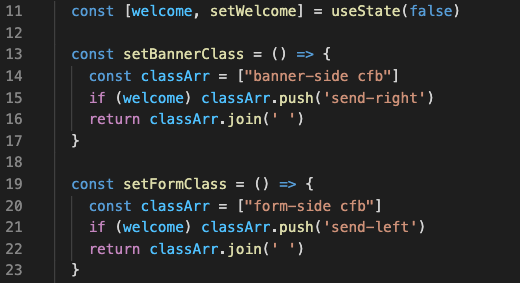


My useState
hook contains a boolean called welcome
. When welcome
is updated, the component will re-render and the classes will be updated based based on that boolean.
我的useState
挂钩包含一个名为welcome
的布尔值。 更新welcome
,该组件将重新呈现,并且基于该布尔值将更新类。
Finally, I put up a button in the banner to trigger setWelcome()
in the useState
hook.
最后,我在横幅中放置一个按钮以触发useState
挂钩中的useState
setWelcome()
。

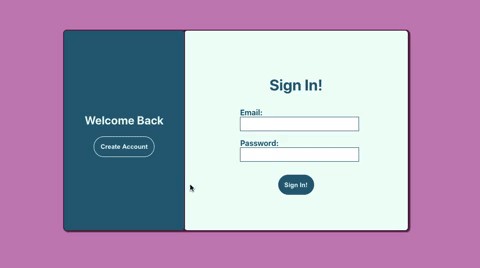
有条件地渲染登录/注册 (Conditionally Render Sign-In / Sign-Up)
The final touch is to change the forms and and banner text based on whether our user intends to sign-in or create a new account. To do this, I used the same welcome
from my useState
hook.
最后一步是根据我们的用户是否打算登录或创建新帐户来更改表单和标题文本。 为此,我使用了useState
挂钩中的相同welcome
useState
。

Because this is a dummy project, I didn’t do anything specific with the forms themselves. If you’d like, you can check them out on GitHub.
因为这是一个虚拟项目,所以我对表单本身没有做任何特定的事情。 如果愿意,可以在GitHub上检出它们。
结论 (Conclusion)
This was a fun one to figure out. The newest concept for me was a deeper understanding of position
and z-index
.
这是一个有趣的发现。 对我来说,最新的概念是对position
和z-index
的更深入的了解。
I’ve used this properties before, but I had been flying by the seat of my pants. I’m happy for my newfound confidence with them.
我以前曾使用过此属性,但我一直在裤子旁边放飞。 我为我对他们的新信心感到高兴。
Taking this project further, I would also animate the rendering of the text and forms themselves. A smooth transition would improve the page.
进一步推进该项目,我还将为文本和表单本身的动画制作动画。 平稳过渡将改善页面。
As always, thanks for reading. I hope this helped you somehow.
与往常一样,感谢您的阅读。 希望这对您有所帮助。
翻译自: https://medium.com/swlh/build-this-cool-sign-in-form-with-react-and-css-f9339d14d140