vue.js 全局应用js
If you’ve ever used Google’s Pagespeed Insights or Google Lighthouse then you’ve seen this webpage performance assessment:
如果您曾经使用过Google的Pagespeed Insights或Google Lighthouse,那么您已经看到了此网页效果评估:
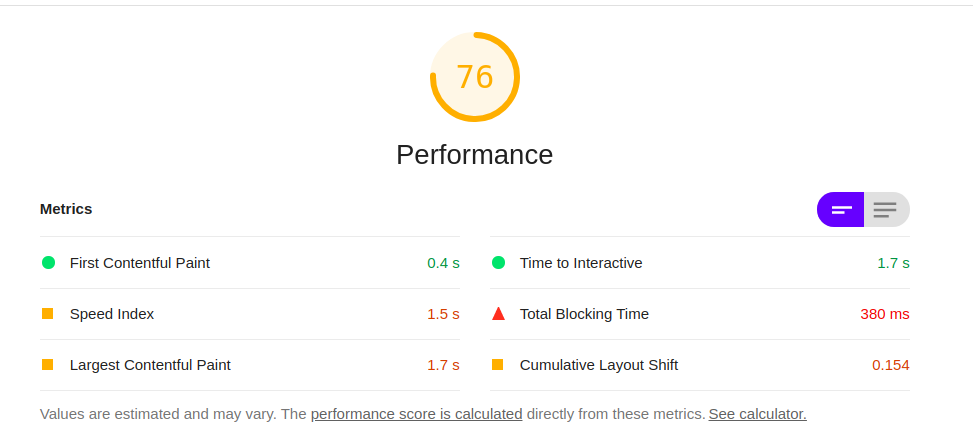
You receive a set of statistics, then some diagnostics on how fast (or slow) your website is.
您会收到一组统计信息,然后会得到一些有关网站速度(或速度)的诊断信息。
Some of the diagnostics can be pretty useful, indicating some easy fixes, unused files, and requests that are slow or take a lot of space.
一些诊断程序可能非常有用,它指示一些简单的修复程序,未使用的文件以及缓慢或占用大量空间的请求。
Other diagnostics, such as main-thread work and Javascript execution time do show problems but they don’t really help you fix them.
其他诊断(例如主线程工作和Javascript执行时间)确实显示了问题,但它们并不能真正帮助您解决问题。
In this article I’ll go through the steps you can follow to make sure your Vue application is working as fast as possible. With these steps, you’ll know exactly what to fix and you won’t have to guess anything.
在本文中,我将按照您可以遵循的步骤进行操作,以确保您的Vue应用程序尽快运行。 通过这些步骤,您将确切地知道要解决的问题,而不必猜测任何事情。
1.仅更新所需内容 (1. Update Only What’s Needed)
One of the nastiest issues you can run into with VueJS is rendering the same elements or list of elements more times than needed. To understand why or how this can happen we have to understand reactivity in Vue.
VueJS可能会遇到的最讨厌的问题之一是渲染相同的元素或元素列表的次数比需要的次数更多。 要了解为什么会发生或如何发生这种情况,我们必须了解Vue中的React性。
This example is from the official Vue.js documentation and it shows which properties are reactive and which are not. There are many reactive elements in Vue: properties assigned to the data object, computed properties, or methods that rely on reactive properties.
该示例来自官方的Vue.js文档,它显示了哪些属性是React性的,哪些不是。 Vue中有许多React式元素:分配给数据对象的属性,计算的属性或依赖于React式属性的方法。
var vm = new Vue({
data: {
a: 1
}
})
// `vm.a` is now reactive
vm.b = 2
// `vm.b` is NOT reactive
But a plain JavaScript code, such as {{ 'value' }}
or {{ new Date() }}
, is not tracked by Vue as a reactive property.
但是Vue不会将普通JavaScript代码(例如{{ 'value' }}
或{{ new Date() }}
作为响应属性进行跟踪。
So what does reactivity have to do with duplicate rendering?
那么React性与重复渲染有什么关系?
Let’s say you have an array of objects like this in your data
object:
假设您的data
对象中有一个像这样的对象数组:
values: [{id: 1, t: 'a'}, {id: 2, t: 'b'}]
And you’re rendering it using v-for
:
然后使用v-for
渲染它:
<div v-for="value in values" :key="value.id">{{ value.t }}</div>
When a new element is added to the list Vue will re-render the whole list. Not convinced? Try writing it like this:
将新元素添加到列表后,Vue将重新渲染整个列表。 不服气吗? 尝试这样写:
<div v-for="value in values" :key="value.id">
{{ value.t }}
{{ new Date() }}
</div>
The JavaScript Date
object is not reactive so it doesn’t affect rendering. It will only be called if the element has to be rendered again. What you will see in this example, is that every time a value is added or removed from values
a new Date will pop up on all rendered elements.
JavaScript Date
对象不是React性的,因此不会影响渲染。 仅当必须再次渲染元素时才调用它。 在此示例中,您将看到,每次在值中添加或删除values
时,所有呈现的元素上都会弹出一个新的Date。
What should you expect in a more optimized page? You should expect only the new or changed elements to show a new Date
, while the others should not be rendered at all.
您应该在更优化的页面中期望什么? 您应该只希望新的或更改的元素显示新的Date
,而其他元素则根本不应该呈现。
So, what does key
do and why are we passing it? The key
property helps Vue understand which element is which. If the order of the array changes, key
helps Vue shuffle the elements into place, rather than going through them one-by-one again.
那么, key
什么,为什么我们要通过它呢? key
属性有助于Vue了解哪个元素是哪个元素。 如果数组的顺序发生变化,则key
可以帮助Vue将元素重新排列到适当的位置,而不是一次又一次地遍历它们。
Specifying a key
is important, but it’s not enough. In order to make sure you’re getting the best performance, you need to create Child
components. That’s right — the solution is pretty simple. You just have to divide your Vue app into small, lightweight components.
指定key
很重要,但这还不够。 为了确保获得最佳性能,您需要创建Child
组件。 没错-解决方案非常简单。 您只需要将Vue应用划分为轻巧的小组件即可。
<item :itemValue="value" v-for="item in items" :key="item.id"></item>
This item component will only update if the specific item has a reactive change (for example with Vue.set)
仅当特定项目具有React性更改(例如,使用Vue.set )时,此项目组件才会更新。
The performance gain of using components to render lists is tremendous. If an element is added or removed from the array, Vue won’t render all the components one-by-one again. If the array is sorted, Vue just shuffles the elements by relying on the provided key
.
使用组件呈现列表的性能提升是巨大的。 如果将元素添加到数组中或从数组中删除,Vue不会再次逐一渲染所有组件。 如果对数组进行了排序,则Vue会依靠提供的key
随机排列元素。
If the component is really lightweight, you can do one better. Instead of passing a complete object like value
you could pass a primitive property (String or Number) like :itemText="value.t”
. With that in place, <item>
will only be re-rendered if the primitive values you passed have changed. This means that even reactive changes will cause an update — but it’s not needed!
如果组件确实很轻巧,则可以做得更好。 而是通过一个完整的对象一样的value
,你可以通过一个基本属性(字符串或数字),如:itemText="value.t”
。随着到位, <item>
只会被重新渲染,如果你传递的原始值有这意味着即使是被动更改也将导致更新-但这不是必需的!
2.消除重复渲染 (2. Eliminate Duplicate Rendering)
Rendering full lists or heavy elements more times than needed is quite a sneaky issue, but it’s very easy to make happen.
渲染完整列表或繁重元素比所需次数更多是一个偷偷摸摸的问题,但很容易实现。
Let’s say you have a component that has an entities
property in the data object.
假设您有一个组件,该组件在数据对象中具有一个entities
属性。
If you followed the previous step, then you probably have a child component to render each entity.
如果遵循上一步,则可能有一个子组件可以呈现每个实体。
<entity :entity="entity" v-for="entity in entities" :key="entity.id" />
The entity template looks something like this:
实体模板看起来像这样:
<template>
<div>
<div>{{ user.status }}</div>
<div>{{ entity.value }}</div>
</div>
</template>
It outputs entity.value
, but it also uses user
from the vuex
state. Now let’s say you have an auth
function that refreshes the user token, or the global user property is changed in any way. This would cause the entire view to update any time something changes, even if user.status
stays the same!
它输出entity.value
,但它也使用来自vuex
状态的user
。 现在,假设您具有一个auth
函数,该函数刷新用户令牌,或者以任何方式更改了全局user属性。 即使user.status
保持不变,这也会导致整个视图在任何更改时更新。
There are a few ways to handle it. A simple one would be to pass the user.status
value as a userStatus
prop from the parent. If the value is unchanged, Vue will not render it again since it doesn’t have to.
有几种处理方法。 一种简单的方法是从父级传递user.status
值作为userStatus
道具。 如果该值保持不变,则Vue不会再次渲染它,因为它不必这样做。
The key here is to be aware of the rendering dependencies. Any prop, data value, or computed value that changes can cause a re-render.
这里的关键是要注意渲染依赖性。 任何更改的属性,数据值或计算值都可能导致重新渲染。
How can you identify duplicate renders?
如何识别重复的渲染?
First of all download the official Vue.js dev tools!
首先下载官方的Vue.js开发工具 !
Then use the “Performance tab” to measure your Vue app’s performance. Press start and stop to run a performance check, ideally during the app load.
然后使用“性能”标签衡量Vue应用的性能。 按开始和停止以运行性能检查,最好在应用程序加载期间。
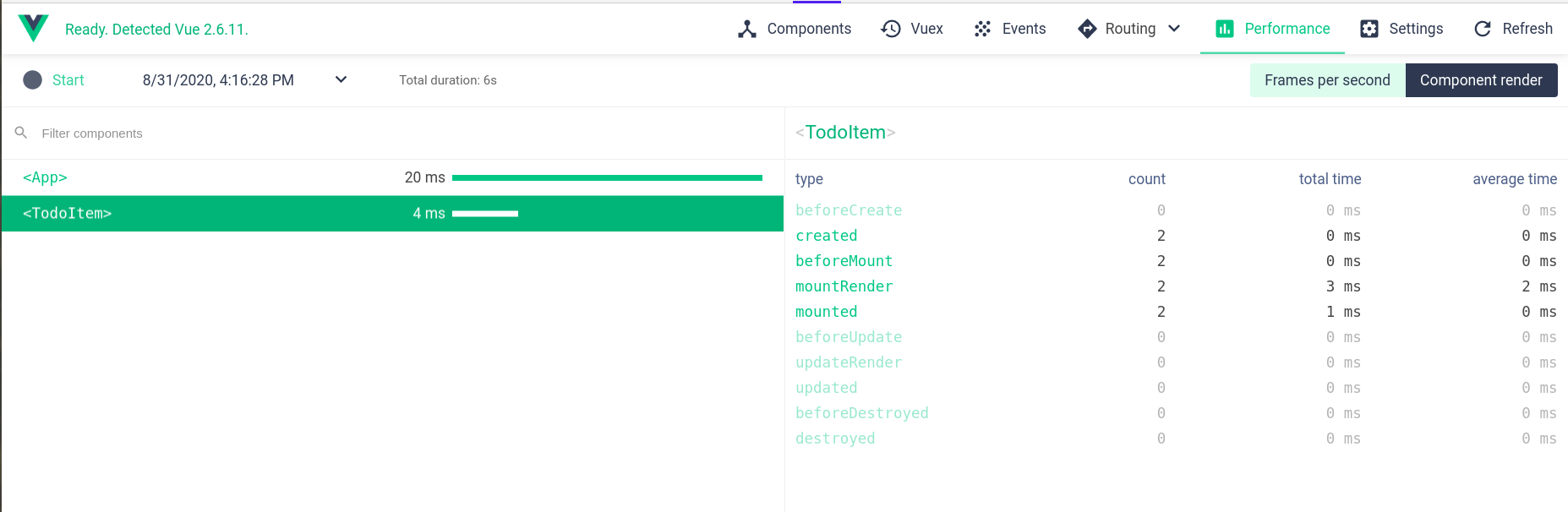
And then go to the “Component Render” tab. If your page renders 100 components, then you should see 100 created
events.
然后转到“组件渲染”选项卡。 如果您的页面呈现100个组件,则应该看到100个created
事件。
What’s important to note here are the updated
events. If you have more updated events than created events, without an update in the actual values, it’s likely you have duplicate changes that lead to duplicate rendering.
这里要注意的是updated
事件。 如果更新后的事件比创建的事件多,而实际值没有更新,则可能是重复的更改导致重复的渲染。
This can happen if your code changes the entities
value many times instead of batching the updates. Or it can happen if you’re using some sort of realtime database like Firestore and you’re getting more snapshots than expected. (Firestore can send many snapshots per update, especially if you have Firestore triggers that update related documents).
如果您的代码多次更改entities
值而不是分批更新,则可能会发生这种情况。 或者,如果您使用诸如Firestore之类的实时数据库,并且快照数量超出预期,则可能会发生这种情况。 (Firestore每次更新可以发送许多快照,特别是如果您具有用于更新相关文档的Firestore触发器时)。
The solution here is to avoid changing entities more times than is necessary. In the case of Firestore snapshots, you can use debounce or throttle functions to avoid changing the entities
property too often.
此处的解决方案是避免多次更改实体。 对于Firestore快照,您可以使用反跳或调节功能来避免过于频繁地更改entities
属性。
3.优化事件处理 (3. Optimize Event Handling)
Not all events are created equal and as such you have to make sure you’re correctly optimizing for each of them.
并非所有事件都是平等创建的,因此必须确保为每个事件正确进行了优化。
Two great examples are @mouseover
and window.scroll
events. These two types of events can be triggered many times even with normal usage. If the event handlers you have in place make costly calculations, these calculations will run multiple times per second causing lag in your application. A solution is to use a debounce function to limit how many times you process these events.
两个很好的例子是@mouseover
和window.scroll
事件。 即使正常使用,这两种类型的事件也可以触发多次。 如果您使用的事件处理程序进行了昂贵的计算,这些计算将每秒运行多次,从而导致应用程序出现滞后。 一种解决方案是使用反跳功能来限制处理这些事件的次数。
4.删除或减少慢速组件 (4. Remove or Reduce Slow Components)
When creating new components yourself, but especially when importing third-party components, you need to make sure they perform well.
自己创建新组件时,尤其是在导入第三方组件时,需要确保它们的性能良好。
You can use the Vue.js dev tools performance tab to estimate the rendering time of each component you’re using. When adding a new component, you can see how much time it takes to render in comparison with your own components.
您可以使用Vue.js开发工具的“性能”标签来估算所使用的每个组件的渲染时间。 添加新组件时,与自己的组件相比,您可以看到渲染所需的时间。
If the new component takes considerably more time than your own components, then you may need to look at alternative components, remove it, or try to reduce its usage.
如果新组件比您自己的组件花费更多的时间,那么您可能需要查看替代组件,将其删除或尝试减少其使用量。
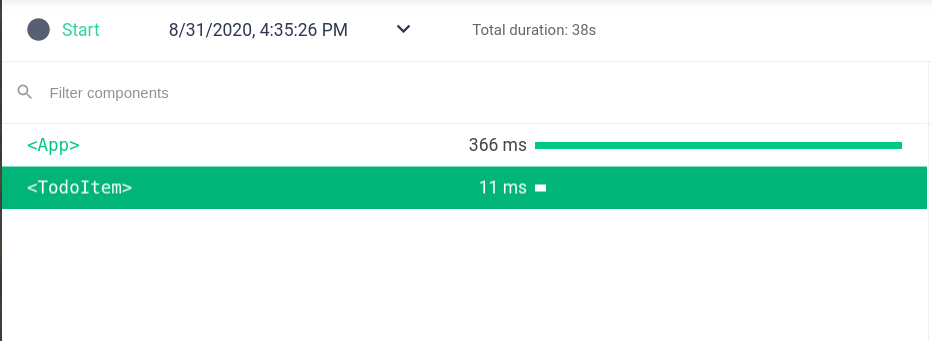
5.渲染一次 (5. Render Once)
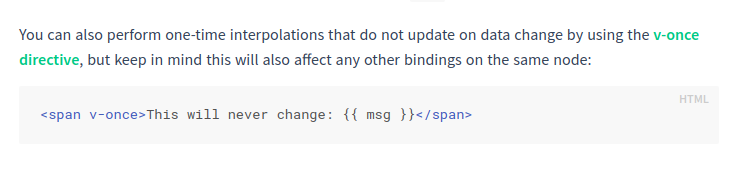
This is from the official Vue.js documentation. If you have elements that once everything is mounted should only be rendered once, you can use the v-once directive.
这来自官方的Vue.js文档。 如果您拥有一旦安装完所有内容后仅应渲染一次的元素,则可以使用v-once指令。
Let’s say you have a section in your app that requires that data does not change for the entire session. By using the v-once directive you ensure this section is only rendered once.
假设您的应用程序中有一个部分,要求整个会话的数据均不得更改。 通过使用v-once指令,您可以确保此部分仅呈现一次。
6.虚拟滚动 (6. Virtual Scrolling)
This is the final step towards optimizing your Vue app performance. Have you ever scrolled Facebook on desktop? (Or Twitter, or Instagram, or any popular social media app?) I bet you have! You can scroll endlessly, without the page slowing down. But if you have a huge list to render in your Vue app you will see the page slowing down the longer the page becomes.
这是优化Vue应用性能的最后一步。 您曾经在台式机上滚动过Facebook吗? (或者Twitter,Instagram或任何流行的社交媒体应用程序?)我敢打赌,你有! 您可以无限滚动,而不会降低页面速度。 但是,如果您要在Vue应用程序中渲染的列表很大,则页面变长的时间就会变慢。
If you decide to implement endless scrolling instead of paging, you can and should use one of these two open-source projects for virtual scrollers and rendering huge lists of items:
如果决定实现无穷滚动而不是分页滚动,则可以并且应该将这两个开源项目之一用于虚拟滚动条并呈现大量项目:
https://github.com/tangbc/vue-virtual-scroll-list
https://github.com/tangbc/vue-virtual-scroll-list
https://github.com/Akryum/vue-virtual-scroller
https://github.com/Akryum/vue-virtual-scroller
I have used both and in my experience vue-virtual-scroll-list
is better because it’s easier to use and doesn’t rely on absolute positions that can break the UI.
我曾经使用过,根据我的经验, vue-virtual-scroll-list
更好,因为它更易于使用并且不依赖于会破坏UI的绝对位置。
结论 (Conclusion)
Even though this guide doesn’t account for all scenarios, these six ways cover a lot of common performance issues for Vue applications.
即使本指南并未涵盖所有情况,但这六种方式仍涵盖了Vue应用程序的许多常见性能问题。
Achieving great front end performance is more important than you may realize. Developers usually have much better computers than the users who end up using your app. Your users may not even use computers but rather slow and outdated smartphones.
实现出色的前端性能比您可能意识到的更为重要。 开发人员通常拥有比最终使用您的应用程序的用户更好的计算机。 您的用户甚至可能不使用计算机,而使用速度较慢且过时的智能手机。
So it’s up to us, the developers, to do the best in our power and deliver an optimal user experience. You can use these six ways as a checklist to help ensure your Vue app is running smoothly for all users.
因此,开发人员有责任尽我们最大的努力并提供最佳的用户体验。 您可以将这六种方法用作检查清单,以帮助确保您的Vue应用程序对所有用户流畅运行。
翻译自: https://medium.com/better-programming/6-ways-to-speed-up-your-vue-js-application-2673a6f1cde4
vue.js 全局应用js