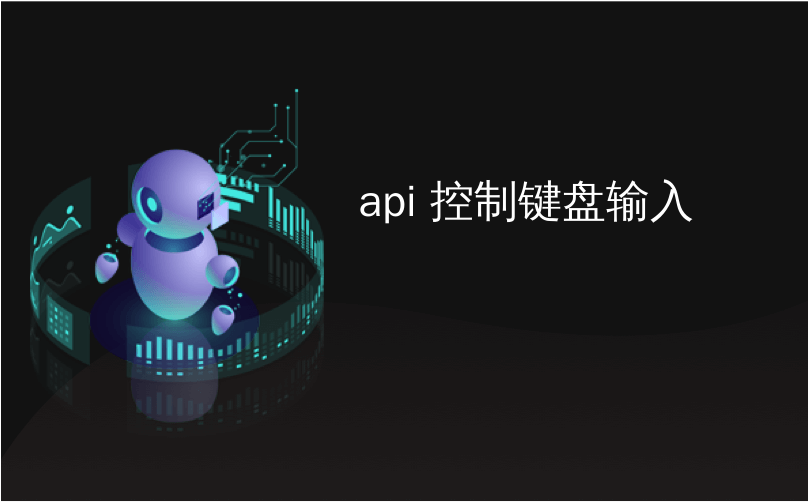
api 控制键盘输入
Every once in a while I feel inspired to create a little tool to "do one thing" (tm). But often I get distracted and a little too lazy to get off the ground and forget all about it. So I thought maybe a little helper can, well, help move things along.
每隔一段时间,我都会被启发去创建一个“做一件事情”(tm)的小工具。 但是,我常常会分心,懒得离开地面,忘记一切。 所以我认为也许有一点帮手可以帮助推动事情发展。
Enter FAIL, short for File API Input Layer (yup, totally made up to match the acronym).
输入FAIL ,它是文件API输入层的缩写(是的,完全由首字母缩写组成)。
失败 (FAIL)
It's a very, very simple blueprint for any single-page tool that needs to read a file (or files) from the user and do something with this file. All client-side, naturally, what cannot be done in JavaScript these days?
对于需要从用户读取一个文件(或多个文件)并对该文件执行某些操作的任何单页工具来说,这都是非常非常简单的蓝图。 自然,所有客户端这些天用JavaScript无法完成什么?
Here's the thing in action - it takes images through drag and drop or though a file input dialog. Then simply shows the images with some data about them:
这就是实际的情况-它通过拖放或通过文件输入对话框来获取图像。 然后简单地显示带有一些数据的图像:
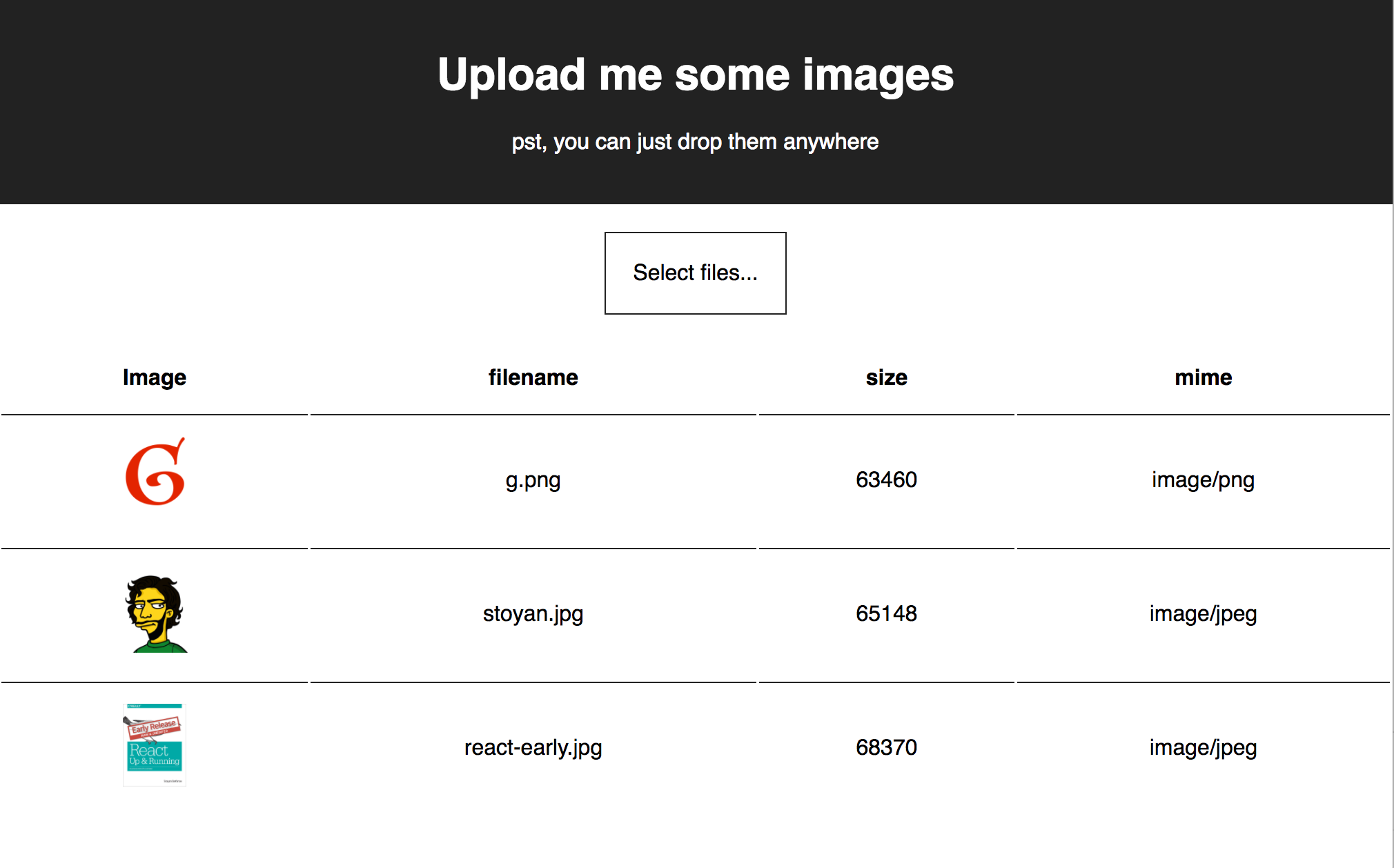
FAIL doesn't do anything with the images, it's the job of the future tools that could be using it.
FAIL对图像不做任何事情,这是将来可能会使用它的工具的工作。
DEMO
演示
React(React)
FAIL is written in React. I'm probably still a little old-school and when I have an idea I create a blank test.html and go from there, vanilla-like. But in this case I decided to go against my lazy-bum instinct and use something that can get me off the ground. And allow me to write all the ES2019 I want. Even though this means dreaded SETUP. I hate setting stuff up, kills the mood 🙂 But in this case turns out React is just perfect for this type of tool.
FAIL用React编写。 我可能还有些老套,当我有一个主意时,我创建了一个空白的test.html,然后从那里开始,就像香草一样。 但是在这种情况下,我决定违背我的懒惰本能,并使用可以使我脱胎换骨的东西。 并允许我编写我想要的所有ES2019。 即使这意味着可怕的设置。 我讨厌设置东西,扼杀了心情🙂但是在这种情况下,React恰恰是这种工具的完美选择。
I couldn't be bothered with Redux or whatever though, not even my self-grown DIY flux implementation. That would be too much.
我对Redux或其他任何东西都不会感到困扰,甚至连我自己开发的DIY流量实现也不会。 那太过分了。
I used create-react-app
to get started:
我使用create-react-app
入门:
$ create-react-app fail
$ cd fail
$ npm start
码(Code)
I shoved all the JS in one file (can't be bothered) and it still ended up under 100 lines of code. The app's components are composed like:
我把所有的JS都塞进了一个文件中(不能打扰),但是它仍然以100行代码结尾。 该应用程序的组件组成如下:
<App>
<Uploads />
<Results />
</App>
App
is actually the one generated by create-react-app
. In its render()
I put:
App
实际上是由create-react-app
。 在其render()
我输入:
render() {
return (
<div className="App">
<div className="App-header">
<h1>Upload me some images</h1>
<p>pst, you can just drop them anywhere</p>
</div>
<div className="Tool-in">
<Uploads onChange={this.handleUploads.bind(this)} />
</div>
<div className="Tool-out">
<Results files={this.state.files} />
</div>
</div>
);
}
Simple, eh?
简单吧?
Now Uploads
and Results
are even simpler. They just render something. They don't need to maintain state. So they can be implemented as stateless functional components. If you're not familiar with those see this diff where I switched from ES class syntax to functional components.
现在, Uploads
和Results
变得更加简单。 他们只是渲染东西。 他们不需要维持状态。 因此它们可以实现为无状态功能组件。 如果您不熟悉这些内容,可以从我从ES类语法切换到功能组件的比较中看到。
Uploads
is just a file input:
Uploads
只是文件输入:
const Uploads = ({onChange}) =>
<div>
<label htmlFor="files" className="Uploads-select">Select files...</label>
<input
type="file"
id="files"
multiple
accept="image/*"
style={{display: 'none'}}
onChange={onChange}
/>
</div>;
Results
just loops though the uploaded files to put up a table:
Results
只是循环通过上传的文件来建立一个表:
const Results = ({files}) => {
if (files.length === 0) {return <span/>;}
return (
<table className="Results-table">
<tbody>
<tr><th>Image</th><th>filename</th><th>size</th><th>mime</th></tr>
{files.map((f, idx) => {
if (!f.type.startsWith('image/')) {
return null;
}
return (
<tr key={idx}>
<td><img alt={f.name} src={window.URL.createObjectURL(f)} height="60" /></td>
<td>{f.name}</td>
<td>{f.size}</td>
<td>{f.type}</td>
</tr>
);
})}
</tbody>
</table>
);
}
Finally the "brains" or the non-render methods of the App
component:
最后是App
组件的“大脑”或非渲染方法:
constructor() {
super();
this.state = {
files: [],
};
document.documentElement.ondragenter = e => e.preventDefault();
document.documentElement.ondragover = e => e.preventDefault();
document.documentElement.ondrop = e => {
e.preventDefault();
this.update(e.dataTransfer.files); // dropped files
}
}
handleUploads(e) {
this.update(e.target.files); // from file input
}
update(moreFiles) {
const files = Array.from(moreFiles);
if (!files) {
return;
}
this.setState({
files: this.state.files.concat(files)
});
}
As you can see all we need to do is maintain the list of files
in the state
and all comes to place.
如您所见,我们需要做的就是保持state
中的files
列表,所有files
就位。
The constructor also takes care of setting up drag-drop listeners.
构造函数还负责设置拖放侦听器。
祝你好运! (C'est tout!)
Once again - code and demo.
再次进行代码和演示。
I'd be thrilled if anyone uses this as a jumping point to create different tools. We can never have too many tools!
如果有人将其用作创建其他工具的起点,我会感到非常兴奋。 我们永远不能拥有太多工具!
Oh yeah - and if you want to learn React in 2017 just buy my book 🙂
哦-是的,如果您想在2017年学习React,请买我的书🙂
Update: Part 2 where the app becomes a PWA
更新:第2部分,应用程序成为PWA
Tell your friends about this post on Facebook and Twitter
在Facebook和Twitter上告诉您的朋友有关此帖子的信息
翻译自: https://www.phpied.com/fail/
api 控制键盘输入