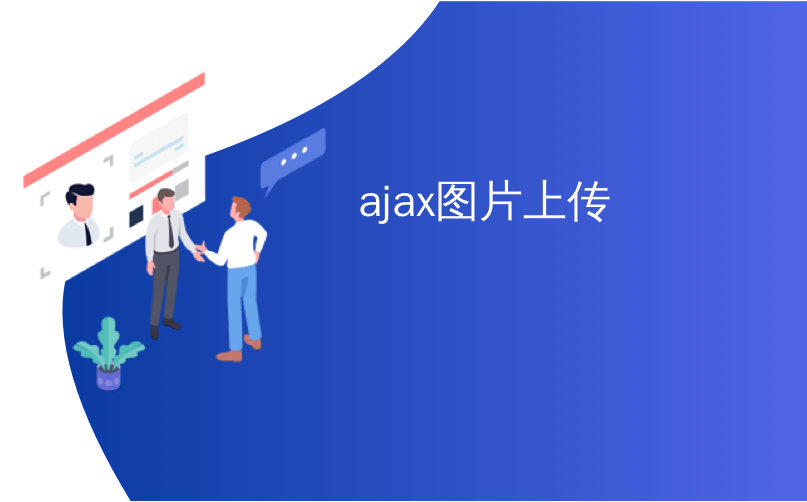
ajax图片上传
So you can do Ajaxy stuff with XMLHttpRequest
or or iframes or dynamic JavaScript tags or... how about simple images. This is best used for simple stuff where you can have a limited number of predefined responses, such as "success" and "oops".
因此,您可以使用XMLHttpRequest
或iframe或动态JavaScript标记或...处理Ajaxy东西,简单图像如何。 这最适用于简单的事物,在这些事物中您可以具有有限数量的预定义响应,例如“成功”和“哎呀”。
All you do is create an image and set its source and this makes a request:
您要做的就是创建一个图像并设置其来源,然后发出一个请求:
new Image().src = "mystuff.php";
This is if you don't care about the response. If you want to inspect the response though you can attach onload
and onerror
handlers:
这是如果您不关心响应。 如果您想检查响应,但是可以附加onload
和onerror
处理程序:
var i = new Image();
i.onload = function() {
// inspect the response
};
i.src = "mystuff.php";
If you can assume you'll have "OK" response most of the time, you can have the .php return a 204 No Response
which is the smallest response (no body). If the .php determines there's something wrong, it then can return an image.
如果您可以假设大多数情况下都会收到“ OK”响应,则可以让.php返回204 No Response
,这是最小的响应(没有body )。 如果.php确定有问题,则可以返回图像。
When you send a 204 response, the onerror
handler will be called because the response is not really an image. It looks backwards to have your success handler be called onerror, but if you expect more successes than errors, then it's probably worth it.
当您发送204响应时,将调用onerror
处理函数,因为该响应实际上不是图像。 将您的成功处理程序称为onerror,向后看,但是如果您期望成功比错误更多,那么可能值得。
var i = new Image();
i.onload = function() {
// an error occurred
};
i.onerror = function() {
// success!
};
i.src = "mystuff.php";
And the final thing - if you want to have coded responses, in other words be able to differentiate between different errors (each with its error code), you can have the .php return different image sizes. Say with constant height but varying width. E.g. 1x1 image, 2x1, 3x1 and so on. In the onload
you inspect the size of the image and determine the type of response.
最后一件事-如果您希望获得编码响应,换句话说,能够区分不同的错误(每个错误均带有错误代码),则.php可以返回不同的图像大小。 以恒定的高度说但宽度可变。 例如1x1图片,2x1、3x1等。 在onload
您检查图像的大小和确定响应的类型。
var i = new Image();
i.onload = function() {
// an error occurred
if (i.width === 1) {
// error #1
}
if (i.width === 7) {
// error #7
}
// etc...
};
i.onerror = function() {
// success!
};
i.src = "mystuff.php";
I'm using a different errors as an example, but you can always have it the other way around: you consider onload a suceess and there are different types of successes.
我以一个不同的错误为例,但是您总是可以用另一种方式解决它:您考虑将加载成功,并且成功的类型多种多样。
电子邮件地址验证示例 (Email address validation example)
Let's take a look at a little more practical example. Let's validate email addresses on the server.
让我们看一个更实际的例子。 让我们验证服务器上的电子邮件地址。
We'll return 7 different image sizes if the supplied email address is invalid or a 204 response is the email looks fine.
如果提供的电子邮件地址无效或204响应是正常的电子邮件,我们将返回7种不同的图像尺寸。
The OK response:
好的响应:
function ok() {
header("HTTP/1.0 204 No Content");
}
The error response that generates an image with a desired width and height of 1 px:
生成具有所需宽度和高度为1 px的图像的错误响应:
function image($width) {
header("Content-Type: image/png");
$im = imagecreate($width, 1);
imagecolorallocate($im, 0, 0, 0);
imagepng($im);
imagedestroy($im);
die();
}
The error codes:
错误代码:
// 1x1 = empty input
// 2x1 = missing @
// 3x1 = too many @s
// 4x1 = missing username
// 5x1 = missing host
// 6x1 = blocked
// 7x1 = no dns record for host
// 204 = OK
And, finally, the "business" logic:
最后,“业务”逻辑:
$email = (string)@$_GET['email'];
if (!$email) {
image(1);
}
// split to username and domain
$splits = explode('@', $email);
if (count($splits) === 1) {
image(2);
}
if (count($splits) !== 2) {
image(3);
}
list($user, $host) = $splits;
if (!$user) {
image(4);
}
if (!$host) {
image(5);
}
$blocked = array('yahoo.com', 'gmail.com', 'hotmail.com');
if (in_array($host, $blocked)) {
image(6);
}
if (!checkdnsrr($host)) {
image(7);
}
// all fine, 204
ok();
测试页 (Test page)
You can play with the test page here: http://www.phpied.com/files/imaje/test.html
您可以在此处使用测试页面: http : //www.phpied.com/files/imaje/test.html
The markup:
标记:
<input id="i"><button id="b">check email</button><pre id="r">enter an email</pre>
And the JS that makes a requests and checks for ok/error:
以及发出请求并检查正常/错误的JS:
var i;
$('b').onclick = function() {
i = new Image();
i.onerror = function() {
$('r').innerHTML = "thanks!";
};
i.onload = function() {
$('r').innerHTML = "invalid email address!";
};
i.src = "imaje.php?email=" + encodeURIComponent($('i').value);
$('r').innerHTML = "checking...";
};
All there is to it!
一切都有!
详细页面 (Verbose page)
A more verbose test can inspect the width of the returned image and display an appropriate message to the user.
更详细的测试可以检查返回图像的宽度,并向用户显示适当的消息。
Play with it here: http://www.phpied.com/files/imaje/verbose.html
在这里玩: http : //www.phpied.com/files/imaje/verbose.html
var i;
$('b').onclick = function() {
i = new Image();
i.onerror = ok;
i.onload = function() {
err(i.width);
}
i.src = "imaje.php?email=" + encodeURIComponent($('i').value);
$('r').innerHTML = "checking...";
};
function ok() {
$('r').innerHTML = "this is one valid email address, good for you!";
}
function err(num) {
var errs = [
'',
'Seriously, I need an email',
"Where's the @?",
"Too many @s",
"Missing username",
"Missing mail host",
"BLOCKED! Go away!",
"Not a valid mail server",
];
$('r').innerHTML = errs[num];
}
A good side effect of using a global i
is that async responses don't mess up the result. E.g. you send requests #1 and #2, #2 arrives first and is OK, #1 arrives later and is an error. At this point you've overwritten i and the handlers for #1 are no longer available. Dunno is it's possible but it would be cool to be able to further abort a previous request if you've made one after it.
使用全局i
一个好的副作用是异步响应不会弄乱结果。 例如,您发送请求#1和#2,#2首先到达并且确定,#1之后到达并且是错误。 至此,您已经覆盖了i,并且#1的处理程序不再可用。 Dunno是有可能的,但是如果您在之前的请求之后发出请求,可以进一步中止先前的请求将很酷。
谢谢 (Thanks)
Thanks for reading! I know it's hardly new for you, my two faithful readers, but these responses with varying image size came up in a conversation recently and as it turns out there are rumors that there might be about 3 developers in Chibougamau, Quebec, that are not aware of this technique.
谢谢阅读! 我知道,我的两位忠实读者对您来说这并不陌生,但是最近在一次对话中出现了这些图像大小各异的回复,事实证明,有传言说魁北克省奇布加茂可能有大约3个开发人员不知道这种技术。
Tell your friends about this post on Facebook and Twitter
在Facebook和Twitter上告诉您的朋友有关此帖子的信息
翻译自: https://www.phpied.com/ajax-with-images/
ajax图片上传