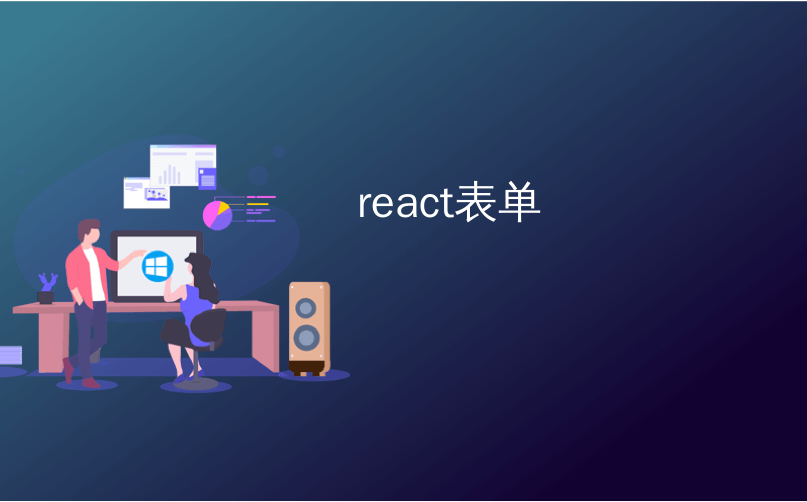
react表单
In the previous post I mentioned the pains, misery and suffering attached to creating and updating an HTML table in DOM land. Now let's see how you do this simple task in React.
在上一篇文章中,我提到了在DOM领域创建和更新HTML表所带来的痛苦,痛苦和痛苦。 现在让我们看看如何在React中完成这个简单的任务。
Demo
演示版
React is all about components. So let's build one.
React是关于组件的。 因此,让我们构建一个。
Let's call it Table
(to avoid any confusion what the component is about).
让我们将其称为Table
(为避免混淆该组件的含义)。
var Table = React.createClass({
/*stuff goeth here*/
});
You see that React components are defined using a regular JS object. Some properties and methods of the object such as render()
have special meanings, the rest is upforgrabs.
您会看到React组件是使用常规JS对象定义的。 对象的某些属性和方法(例如render()
具有特殊含义,其余的都是upforgrabs。
<rant>createClass
should've been named createComponent
, but... naming is hard </rant>
<rant> createClass
应该被命名为createComponent
,但是...命名很困难</ rant>
数据,甜数据 (Data, sweet data)
A table is a way to neatly and orderly present some data. The data is front and center, the most important thing. And data is what you should focus on - retrieving, updating, saving, etc.
表格是一种整齐有序地呈现某些数据的方法。 数据是最重要的,最重要的。 数据是您应关注的重点—检索,更新,保存等。
Once you have the data, you let React take care of the presentation part.
一旦有了数据,就可以让React处理演示部分。
You want the UI to react to changes in the data. So you need a stateful component. Meaning one that has state. This is easier than it sounds.
您希望UI对数据中的更改做出React。 因此,您需要一个有状态的组件。 意思是有状态的。 这比听起来容易。
州 (State)
Here's how to setup the obligatory getInitialState()
method that gives you the initial state of the state.
这是设置必需的getInitialState()
方法的方法,该方法为您提供state的初始状态。
var Table = React.createClass({
getInitialState: function() {
return {data: this.props.data};
}
});
As you can see the state is an object. Simple as that, the old familiar {key: value}
situation. In this case the state is just one property of the state
object, appropriately named data
.
如您所见,状态是一个对象。 如此简单,就是以前熟悉的{key: value}
情况。 在这种情况下,状态只是state
对象的一个属性,可以适当地命名为data
。
The initial state comes from the data
property I decided to use to initialize the component (your components can have properties which the users of the components can define, just like an HTML element can have attributes). This is optional but I think convenient.
初始状态来自我决定用于初始化组件的data
属性(您的组件可以具有组件的用户可以定义的属性,就像HTML元素可以具有属性一样)。 这是可选的,但我认为很方便。
使用组件 (Using the component)
In order to use the new component, you go:
为了使用新组件,您需要执行以下操作:
React.renderComponent(
Table({data: [/*sweet data*/] }),
where);
Here where
is the DOM node you point React to. In an all-react app, this is the only time you touch the DOM yourself. Then you let React run wild!
这里您指向React的DOM节点在where
。 在全React式应用程序中,这是您唯一一次触摸DOM的情况。 然后,让React疯狂运行!
已经渲染 (Render already)
Now React knows about the data and can react to changes in it. The only missing piece is the render()
method each component must implement. As you know all the UI is built entirely in JS.
现在,React知道了数据并且可以对其中的更改做出React。 唯一缺少的部分是每个组件必须实现的render()
方法。 如您所知,所有UI都是完全用JS构建的。
In this case the rendering is about creating table
and tbody
and then looping through the data to create tr
s and td
s. React gives you wrappers to these html elements via the React.DOM.*
functions.
在这种情况下,渲染是关于创建table
和tbody
,然后遍历数据以创建tr
s和td
。 React通过React.DOM.*
函数为您提供了这些html元素的包装。
render: function () {
return (
React.DOM.table(null, React.DOM.tbody(null,
this.state.data.map(function (row) {
return (
React.DOM.tr(null,
row.map(function (cell) {
return React.DOM.td(null, cell);
})
)
);
})
))
);
}
As you see, you use array's map()
to traverse this.state.data
and do rows. Then another map()
does the cells in each row. Easy enough.
如您所见,您使用数组的map()
遍历this.state.data
并执行行。 然后另一个map()
处理每一行中的单元格。 很简单。
现在都在一起了 (All together now)
You put the whole thing in an HTML file.
您将整个内容放入HTML文件中。
<div id="app"></div><!-- app goes here -->
<script src="build/react.min.js"></script> <!-- include React, ~18K gzipped-->
<script>
//
// create component
//
var Table = React.createClass({
getInitialState: function() {
return {data: this.props.data};
},
render: function () {
return (
React.DOM.table(null, React.DOM.tbody(null,
this.state.data.map(function (row) {
return (
React.DOM.tr(null,
row.map(function (cell) {
return React.DOM.td(null, cell);
})
)
);
})
))
);
}
});
//
// get data somehow
//
var data = [[1,2,3],[4,5,6],[7,8,9]];
//
// Render the component in the "app" DOM node
// giving it the initial data
//
var table = React.renderComponent(
Table({data: data}),
app);
</script>
That's all there is to it!
这里的所有都是它的!
更新 (Updates)
Data, like life, changes. What's a dev to do?
数据就像生活一样,在发生变化。 开发人员要做什么?
In React you simply pass the data to the rendered component and forget!
在React中,您只需将数据传递给渲染的组件就可以了!
The component was assigned to the table
var. So you do:
该组件已分配给table
var。 所以你也是:
table.setState({data: [/* up-to-date data */] })
React then takes care of updating the DOM very very efficiently, only where necessary. If only one cell changes, only one is updated!
然后,React会非常高效地(仅在必要时)负责更新DOM。 如果只有一个单元格发生更改,则只会更新一个!
Go to the demo and play in the console, for example:
转到演示并在控制台中播放,例如:
// single cells
data[0][0] = "baba"; table.setState({data: data});
data[1][1] = "bubu"; table.setState({data: data});
// different tables altogether
data = [[5,6],[2,3],[0,0]]; table.setState({data: data});
data = [[5,6,7,6,7,8],[1,1,1,1,2,3],[1,1,1,0,0,0]]; table.setState({data: data});
You can open your console tools and observe what part of the DOM is updated.
您可以打开控制台工具,观察DOM的哪一部分已更新。
还有一件事:JSX (One more thing: JSX)
How about that render()
method, eh? Lots of function calls and parens to close? Enters JSX. Boom! It lets you write the all-familiar HTML (ok, XML) inside the JavaScript.
那个render()
方法怎么样? 有很多函数调用和括号可以关闭吗? 输入JSX。 繁荣! 它使您可以在JavaScript内编写所有熟悉HTML(好的XML)。
Here's how the rendering code will look like when you use JSX:
使用JSX时,呈现代码如下所示:
<table><tbody>
{this.state.data.map(function(row) {
return (
<tr>
{row.map(function(cell) {
return <td>{cell}</td>;
})}
</tr>);
})}
</tbody></table>
Now you obviously need to transform this code to the regular JavaScript calls you saw before. It's surprisingly easy and convenient, but I'll leave it for the next blog post. Meanwhile I leave you with the JSX docs. And remember, JSX is optional (but so darn convenient!), you can write the JS function calls yourself.
现在,您显然需要将此代码转换为之前看到的常规JavaScript调用。 它出奇的简单和方便,但是我将在下一篇博客文章中保留它。 同时,我把JSX文档留给您。 请记住, JSX是可选的(但太方便了!),您可以自己编写JS函数。
Yeah, and the react-to-dom init can look like:
是的,并且dom-to-dom初始化看起来像:
var data = [[1,2,3],[4,5,6],[7,8,9]];
var table = React.renderComponent(
<Table data={data}/>,
app);
Now, that's so much more HTML-y.
现在,更多的是HTML-y。
现在保重,再见 (Take care now, bye-bye then)
And, just not to give you the wrong impression about React's capabilities with my ugly tables, here's a game called Wolfenstein with the rendering layer written in React: you can play here.
而且,只是为了不给您关于我的丑陋表对React功能的错误印象,这是一个名为Wolfenstein的游戏,其中的渲染层是用React编写的:您可以在此处玩。
Tell your friends about this post on Facebook and Twitter
在Facebook和Twitter上告诉您的朋友有关此帖子的信息
翻译自: https://www.phpied.com/reactive-table/
react表单