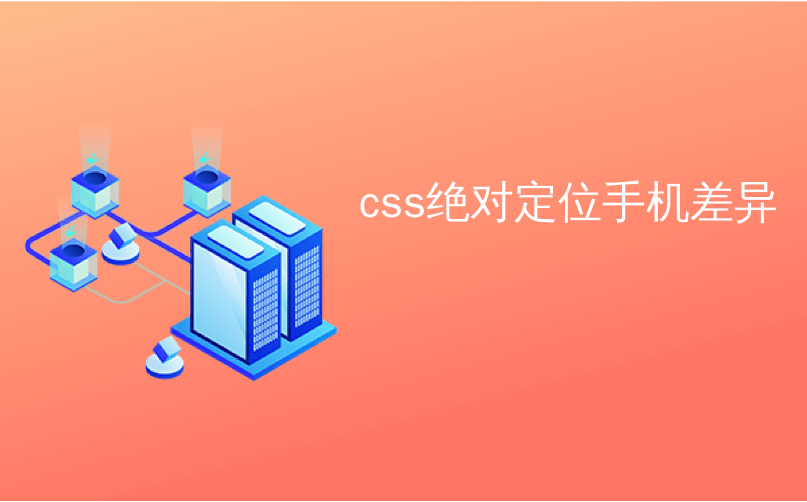
css绝对定位手机差异
Continuing from last night...
从昨晚开始...
First, two twitter responses pointed to even more readily-available options for comparing screenshots. One is Wraith from BBC engineers which supports Firefox/Gecko (via SlimerJS) in addition to PhantomJS. The other is the almost-ready siteeffect.io which is based on http://dalekjs.com/ which seems to support all the browsers!
首先,两个Twitter响应指出了用于比较屏幕截图的更容易获得的选项。 BBC工程师的Wraith之一就是PhantomJS,它还支持Firefox / Gecko(通过SlimerJS)。 另一个是基于http://dalekjs.com/的几乎可以使用的siteeffect.io ,它似乎支持所有浏览器!
Anyway, I thought I should add Firefox support to my brave little script via SlimerJS and also do what I hinted at the end of the previous post - do the image diff in nodejs-land instead of ImageMagick.
无论如何,我认为我应该通过SlimerJS将Firefox支持添加到我勇敢的小脚本中,并且也按照我在上一篇文章末尾的提示进行操作-在nodejs-land而不是ImageMagick中进行图像比较。
SlimerJS (SlimerJS)
It's pretty cool, just like PhantomJS. I didn't manage to install it using regular package managers (like brew) but luckily you can just download it standalone and run from anywhere. So I shoved in ~/Downloads/slimerjs
就像PhantomJS一样,它非常酷。 我没有使用常规的软件包管理器(例如brew)安装它,但是幸运的是,您可以独立下载它并在任何地方运行。 所以我推到~/Downloads/slimerjs
A quick script to add Mozilla/Gecko support to the url2png functionality:
将Mozilla / Gecko支持添加到url2png功能的快速脚本:
var system = require('system');
var url = system.args[1];
var png = system.args[2];
var page = require('webpage').create();
page.viewportSize = { width: 800, height: 600 };
page
.open(url)
.then(function() {
page.render(png);
phantom.exit();
});
Testing:
测试:
$ ~/Downloads/slimerjs/slimerjs url2png-moz.js http://google.com goo.png
This makes a screenshot of Google's homepage into goo.png
这goo.png
Google主页的屏幕截图变成goo.png
Node.js中的图像差异 (Image diff in nodejs)
I found this library called pngparse (hmm, no GD in NodeJS?) which does exactly what I need - reads a png and gives width/height and array of RGBA pixel values, just like image data you'd get from HTML canvas.
我发现了这个名为pngparse的库(嗯, NodeJS中没有GD?),它确实满足我的需要-读取png并提供宽度/高度和RGBA像素值数组,就像从HTML canvas获得的图像数据一样。
So here's a function that simply returns true
if the two images are the same:
所以这是一个函数,如果两个图像相同,则仅返回true
:
function sameImage(image_a, image_b, cb) {
var pngparse = require('pngparse');
pngparse.parseFile(image_a, function(err, a) {
if (err) {
console.log('Where the first file? ' + image_a + ' is not it.');
process.exit(2);
}
pngparse.parseFile(image_b, function(err, b) {
if (err) {
console.log('Where the second file? ' + image_b + ' is not it.');
process.exit(3);
}
// easy stuffs first
if (a.data.length !== b.data.length) {
return cb(false);
}
// loop over pixels, but
// skip 4th thingie (alpha) as these images should not be transparent
for (var i = 0, len = a.data.length; i < len; i += 4) {
if (a.data[i] !== b.data[i] ||
a.data[i + 1] !== b.data[i + 1] ||
a.data[i + 2] !== b.data[i + 2]) {
return cb(false);
}
}
return cb(true);
});
});
}
The benefits of doing this in Node instead of ImageMagick is just speed. (Disclaimer: Not that I tested but...). There's no separate cmd like process to exec()
, there's no weird metrics to calculate, just give up as soon as just one of the R, G or B channels is different between the two images. Skip Alpha. Or even give up as soon as one of the images has more data, meaning different geometry, meaning definitely not the same image.
在Node而不是ImageMagick中执行此操作的好处仅仅是速度。 (免责声明:不是我测试过,而是...)。 exec()
没有单独的cmd like流程,没有可计算的怪异指标,只要两个图像之间的R,G或B通道之一不同就放弃。 跳过Alpha。 甚至在其中一张图像具有更多数据时就放弃,这意味着不同的几何形状,这意味着绝对不是同一幅图像。
最终结果 (End result)
So with a bit of reshuffling and adding support for both webkit and gecko and changing the image diff logic, here's what I ended up with:
因此,经过一些改组并增加了对webkit和gecko的支持并更改了图像差异逻辑,这就是我最终得到的结果:
var exec = require('child_process').exec;
var read = require('fs').readFileSync;
var sprintf = require('sprintf').sprintf;
var write = require('fs').writeFileSync;
var tmp = process.cwd() + '/tmp/';
var html = read('source/zen.html', 'utf8');
var before = html.replace('{{{FILE}}}', '../source/before.css');
var after = html.replace('{{{FILE}}}', '../source/after.css');
write('tmp/before.html', before);
write('tmp/after.html', after);
var phantoms = [
{name: 'webkit', path: 'phantomjs'},
{name: 'moz', path: '~/Downloads/slimerjs/slimerjs'},
];
phantoms.forEach(function(tool) {
var before = 'tmp/before-' + tool.name + '.png';
var after = 'tmp/after-' + tool.name + '.png';
var giff = 'tmp/giff-' + tool.name + '.gif';
var before_cmd = sprintf('%s url2png-%s.js "%s" %s',
tool.path,
tool.name,
'file://'+ tmp + 'before.html',
before);
var after_cmd = sprintf('%s url2png-%s.js "%s" %s',
tool.path,
tool.name,
'file://'+ tmp + 'after.html',
after);
var giff_cmd = sprintf('convert -delay 50 -loop 0 %s %s %s', before, after, giff);
exec(before_cmd, function() {
exec(after_cmd, function() {
sameImage(before, after, function(same) {
if (same) {
console.log('all good in ' + tool.name);
} else {
exec(giff_cmd);
console.log('Bad, bad! Failure in ' +
tool.name + '. See stuff in ' + tmp +
', e.g. ' + giff + ' to help you debug');
process.exit(1);
}
});
});
});
});
You can browse all the files right here: http://www.phpied.com/files/diffcss2/
您可以在这里浏览所有文件: http : //www.phpied.com/files/diffcss2/
下一个? (Next?)
1. I'm pretty sure there's gotta be a way to do animated GIFs with NodeJS so dump ImageMacick completely 2. What about the elephant in the room - IE6-11?
1.我很确定肯定有一种方法可以使用NodeJS制作GIF动画,因此完全转储ImageMacick 2. 2.房间里的大象-IE6-11呢?
Tell your friends about this post on Facebook and Twitter
在Facebook和Twitter上告诉您的朋友有关此帖子的信息
翻译自: https://www.phpied.com/css-diffs-2/
css绝对定位手机差异